Exploring the Role of the Single Ampersand in Member Function Parameters
Within C programming, the syntax of member functions often includes the use of ampersand symbols (&) as ref-qualifiers for non-static member functions. These symbols can influence the behavior of the function call based on the value category of the object being used to invoke it. This article delves into the significance of using a single ampersand (&) in this context.
Specifically, the presence of a single ampersand & after the parameter list of a member function declaration implies that the function will be invoked when the object is an lvalue reference or a regular, non-reference object. This allows the function to be called directly using the object's name or via an lvalue reference. For instance:
class wrap { public: operator obj() const & { ... } // Invocable via lvalue reference };
In contrast, the absence of any ref-qualifier, meaning no ampersand in the declaration, indicates that the function can be invoked regardless of the value category of the object. This means it can be called using either an lvalue or an rvalue reference.
To further understand the distinction, consider the following code snippet:
struct foo { void bar() {} // Function without a ref-qualifier void bar1() & {} // Function with an lvalue reference ref-qualifier void bar2() && {} // Function with an rvalue reference ref-qualifier };
In this example:
- bar can be invoked using both lvalue and rvalue references.
- bar1 can only be invoked using lvalue references or regular objects (i.e., objects without any reference).
- bar2 can only be invoked using rvalue references.
To illustrate this behavior, the following code demonstrates how these functions can be called based on the value category of the object:
foo().bar(); // Always fine foo().bar1(); // Doesn't compile because bar1 requires an lvalue foo().bar2(); foo f; f.bar(); // Always fine f.bar1(); f.bar2(); // Doesn't compile because bar2 requires an rvalue
In summary, the presence of a single ampersand & after the parameter list of a member function declaration indicates that the function can be invoked when the object is an lvalue reference. This provides a means to restrict the invocation of the function based on the value category of the object being used.
The above is the detailed content of What Does a Single Ampersand (&) Mean in C Member Function Parameters?. For more information, please follow other related articles on the PHP Chinese website!
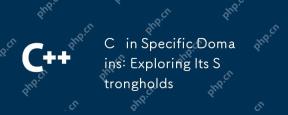
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
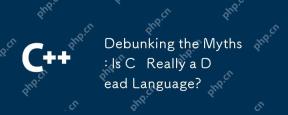
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
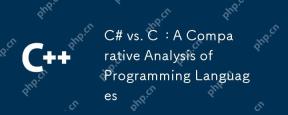
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
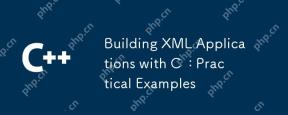
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
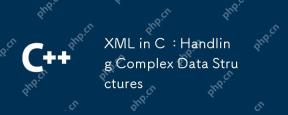
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
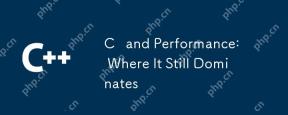
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
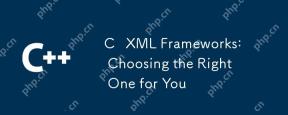
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
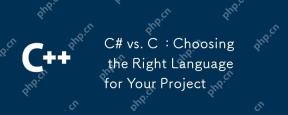
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment
