


How to Convert Multidimensional Arrays into Single Arrays
Problem:
A multidimensional array is encountered with an unnecessary structure. The goal is to simplify it into a single-dimensional array, extracting only the desired values within the nested arrays.
Solution:
To convert a multidimensional array into a single-dimensional array, the array_column() function can be utilized. This function effectively extracts a specific column from a multidimensional array, conceptually similar to extracting a column from a table or matrix.
Implementation:
$array = array_column($array, 'plan');
Breakdown of the Script:
- $array: Represents the multidimensional array that needs conversion.
-
array_column() function: This function takes two arguments:
- The first argument is the multidimensional array to be transformed.
- The second argument identifies the desired column or key to extract. In this case, it specifies 'plan' to retrieve the values for this key.
Example:
Using the provided multidimensional array:
$array = array( 0 => array( 'plan' => 'basic' ), 1 => array( 'plan' => 'small' ), 2 => array( 'plan' => 'novice' ), 3 => array( 'plan' => 'professional' ), 4 => array( 'plan' => 'master' ), 5 => array( 'plan' => 'promo' ), 6 => array( 'plan' => 'newplan' ) );
To convert it into a single-dimensional array, the following code can be used:
$newArray = array_column($array, 'plan');
The resulting $newArray will have the following structure:
Array( [0] => 'basic', [1] => 'small', [2] => 'novice', [3] => 'professional', [4] => 'master', [5] => 'promo', [6] => 'newplan' )
Additional Information:
- For more details on the array_column() function, refer to the official PHP documentation.
- This solution assumes that all inner arrays have the same key structure. If that's not the case, additional processing might be necessary.
The above is the detailed content of How to Flatten a Multidimensional Array in PHP Using `array_column()`?. For more information, please follow other related articles on the PHP Chinese website!
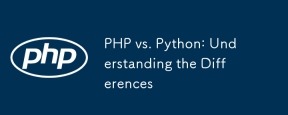
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
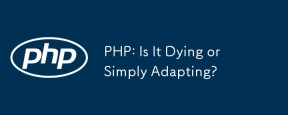
PHP is not dying, but constantly adapting and evolving. 1) PHP has undergone multiple version iterations since 1994 to adapt to new technology trends. 2) It is currently widely used in e-commerce, content management systems and other fields. 3) PHP8 introduces JIT compiler and other functions to improve performance and modernization. 4) Use OPcache and follow PSR-12 standards to optimize performance and code quality.
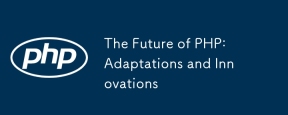
The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
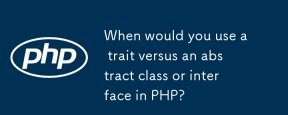
In PHP, trait is suitable for situations where method reuse is required but not suitable for inheritance. 1) Trait allows multiplexing methods in classes to avoid multiple inheritance complexity. 2) When using trait, you need to pay attention to method conflicts, which can be resolved through the alternative and as keywords. 3) Overuse of trait should be avoided and its single responsibility should be maintained to optimize performance and improve code maintainability.
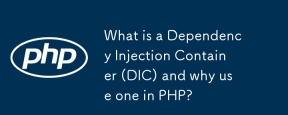
Dependency Injection Container (DIC) is a tool that manages and provides object dependencies for use in PHP projects. The main benefits of DIC include: 1. Decoupling, making components independent, and the code is easy to maintain and test; 2. Flexibility, easy to replace or modify dependencies; 3. Testability, convenient for injecting mock objects for unit testing.
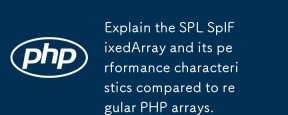
SplFixedArray is a fixed-size array in PHP, suitable for scenarios where high performance and low memory usage are required. 1) It needs to specify the size when creating to avoid the overhead caused by dynamic adjustment. 2) Based on C language array, directly operates memory and fast access speed. 3) Suitable for large-scale data processing and memory-sensitive environments, but it needs to be used with caution because its size is fixed.
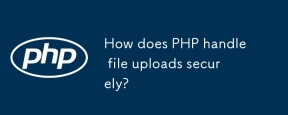
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
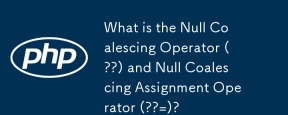
In JavaScript, you can use NullCoalescingOperator(??) and NullCoalescingAssignmentOperator(??=). 1.??Returns the first non-null or non-undefined operand. 2.??= Assign the variable to the value of the right operand, but only if the variable is null or undefined. These operators simplify code logic, improve readability and performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
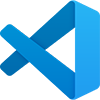
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),