The Necessity of Reference Parameter in Copy Constructors in C
When working with objects in C , it becomes crucial to understand the role of copy constructors, and one essential aspect is passing the parameter by reference. Let's explore the reason why this is mandatory.
Passing Parameter by Reference vs. by Value
In C , parameters can be passed to functions in two ways: by reference or by value. By reference means the function accesses the original variable directly, while by value creates a copy of the original variable for use within the function.
Infinite Recursion with Value Parameter
If the copy constructor were to accept its parameter by value, it would lead to an infinite loop. To understand why, consider the following pseudocode:
class MyClass { int value; public: MyClass(int v) : value(v) {} // Copy constructor with parameter passed by value };
If we call MyClass myObject(5);, the copy constructor will be invoked. However, since the parameter is passed by value, a copy of the original integer is created. To initialize this copy, we need to call the copy constructor again. This leads to an infinite recursion because the copy constructor keeps calling itself without making any progress.
Avoiding Recursion with Reference Parameter
Passing the parameter by reference breaks the infinite recursion. When the parameter is passed by reference, the copy constructor directly operates on the original variable, avoiding the need to create a copy and call the copy constructor recursively.
class MyClass { int value; public: MyClass(int& v) : value(v) {} // Copy constructor with parameter passed by reference };
With the parameter passed by reference, the myObject variable is directly initialized with the value 5 without invoking the copy constructor multiple times.
Conclusion
In summary, the copy constructor's parameter must be passed by reference in C to avoid falling into an infinite recursion. By passing the parameter by reference, the copy constructor can modify the original variable directly, ensuring correct object initialization and preventing unnecessary duplication of work.
The above is the detailed content of Why Must C Copy Constructors Use Reference Parameters?. For more information, please follow other related articles on the PHP Chinese website!
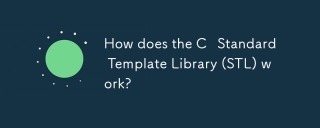
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
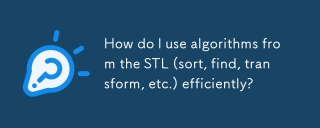
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
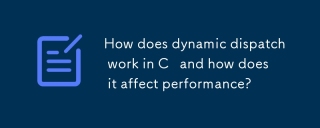
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
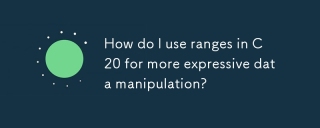
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
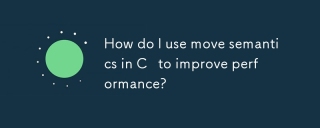
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
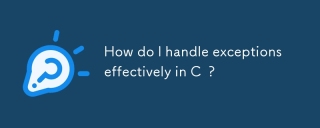
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
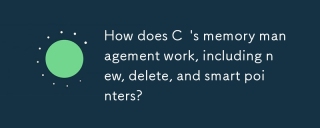
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
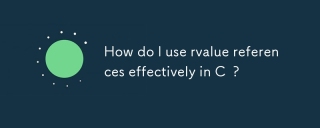
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
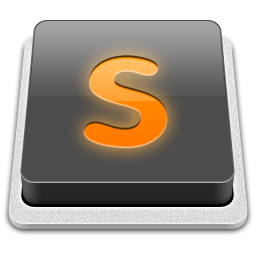
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
