Choosing the Right Controls on an AJAX-Driven Site
Navigating and interacting with AJAX-driven websites can be challenging for automated scripts. Here's a step-by-step guide to effectively automate such websites:
- Observe User Actions: Note down the manual steps you take on the page, including key elements modified or added by JavaScript.
- Identify Page Elements: Use browser tools like Firebug or Chrome Developer Tools to inspect the page structure and determine CSS/jQuery selectors for key elements.
- Manipulate Page Elements: Use jQuery and other browser tools to manipulate static HTML elements. Utilize waitForKeyElements to handle elements dynamically altered by JavaScript or AJAX.
Specific Example: Nike Auto-Purchase Script
Goal: Selecting a specific shoe size, adding it to the cart, and proceeding to checkout on every Nike sneaker page.
Steps:
-
Key Elements Identification:
- Size Dropdown: div.footwear form.add-to-cart-form span.sizeDropdown a.size-dropdown
- Target Shoe Size: a:contains('10')
- Add to Cart Button: div.footwear form.add-to-cart-form div.product-selections div.add-to-cart
- Checkout Button: div.mini-cart div.cart-item-data a.checkout-button:visible
-
Sequence of Events:
- Activate Size Dropdown
- Select Target Shoe Size
- Wait for Shoe Size Display and Add to Cart
- Click the Checkout Button
Complete Script:
// ==UserScript== // @name Nike Auto-Buy Shoes // @include http*://store.nike.com/* // @require http://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js // @require https://gist.github.com/raw/2625891/waitForKeyElements.js // @grant GM_addStyle // ==/UserScript== var targetShoeSize = "10"; //-- Step 1: Activate Size Dropdown waitForKeyElements( "div.footwear form.add-to-cart-form span.sizeDropdown a.size-dropdown", activateSizeDropdown ); function activateSizeDropdown(jNode) { triggerMouseEvent(jNode[0], "mousedown"); //-- Setup Step 2 waitForKeyElements( "ul.selectBox-dropdown-menu li a:contains('" + targetShoeSize + "'):visible", selectDesiredShoeSize ); } //-- Step 2: Select Target Shoe Size function selectDesiredShoeSize(jNode) { if ($.trim(jNode.text()) === targetShoeSize) { //-- Trigger triplex event triggerMouseEvent(jNode[0], "mouseover"); triggerMouseEvent(jNode[0], "mousedown"); triggerMouseEvent(jNode[0], "mouseup"); //-- Setup Steps 3 and 4 waitForKeyElements( "div.footwear form.add-to-cart-form span.sizeDropdown a.selectBox span.selectBox-label:contains('(" + targetShoeSize + ")')", waitForShoeSizeDisplayAndAddToCart ); } } //-- Steps 3 and 4: Wait for Shoe Size Display and Add to Cart function waitForShoeSizeDisplayAndAddToCart(jNode) { var addToCartButton = $( "div.footwear form.add-to-cart-form div.product-selections div.add-to-cart" ); triggerMouseEvent(addToCartButton[0], "click"); //-- Setup Step 5 waitForKeyElements( "div.mini-cart div.cart-item-data a.checkout-button:visible", clickTheCheckoutButton ); } //-- Step 5: Click the Checkout Button function clickTheCheckoutButton(jNode) { triggerMouseEvent(jNode[0], "click"); } function triggerMouseEvent(node, eventType) { var clickEvent = document.createEvent("MouseEvents"); clickEvent.initEvent(eventType, true, true); node.dispatchEvent(clickEvent); }
The above is the detailed content of How Can I Effectively Automate AJAX-Driven Website Interactions?. For more information, please follow other related articles on the PHP Chinese website!
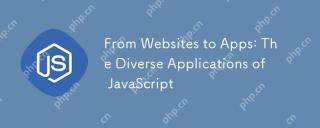
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
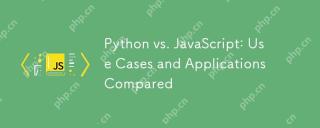
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
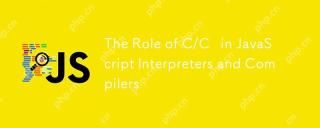
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
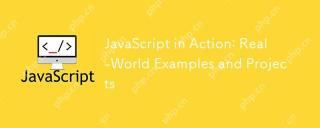
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
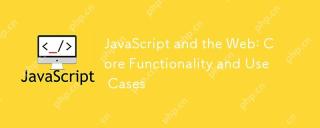
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
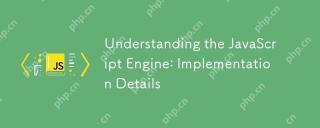
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
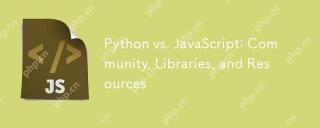
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.