


Detecting Member Variables Using Metaprogramming
Question:
How can we determine whether a class contains a particular member variable, even when its name is unknown or it uses different names in different classes?
Solution:
One approach involves metaprogramming techniques and leverages the decltype operator and SFINAE (Substitution Failure Is Not An Error). Consider the following code:
#include <type_traits> template <typename t typename="int"> struct HasX : std::false_type { }; template <typename t> struct HasX<t decltype t::x> : std::true_type { };</t></typename></typename></type_traits>
Explanation:
- The primary template HasX declares that by default, a class does not have a member variable named x.
- The specialization for U = int overrides this default declaration using SFINAE. When a type T is substituted, it attempts to evaluate (void) T::x. If T has a member variable named x, this expression will succeed, and HasX
derives from std::true_type, indicating that x exists. - The decltype((void) T::x, 0) expression tricks the compiler into treating T::x as an expression of type int. This ensures that SFINAE occurs as expected.
Usage:
To use this technique, declare the template as follows:
template <typename t> bool Check_x(T p, typename HasX<t>::type b = 0) { return true; }</t></typename>
This check would return true for classes with an x member variable, such as:
struct P1 { int x; };
and false for those without, such as:
struct P2 { float X; };
Note:
This solution avoids using C 11 features, such as std::is_member_function_pointer, to maintain compatibility with older compilers.
The above is the detailed content of How Can Metaprogramming Detect the Presence of Member Variables in C Classes?. For more information, please follow other related articles on the PHP Chinese website!
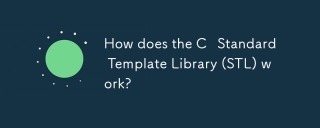
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
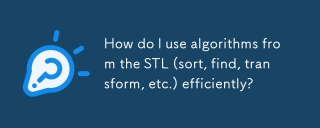
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
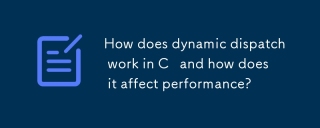
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
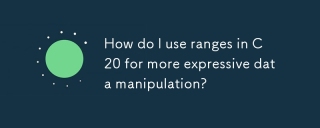
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
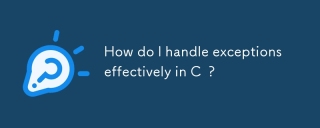
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
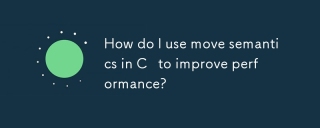
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
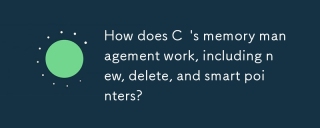
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
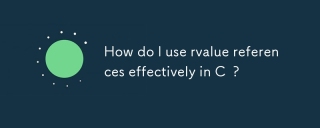
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
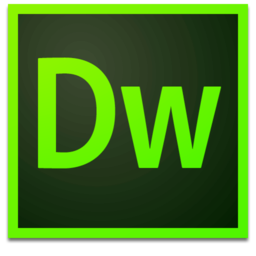
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
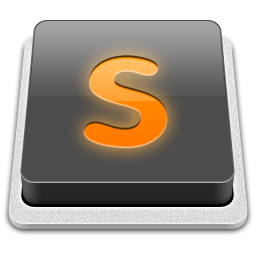
SublimeText3 Mac version
God-level code editing software (SublimeText3)
