Implementing a Map with Multiple Values Per Key
In certain scenarios, it may be necessary to store multiple values under the same key within a HashMap. While Java's standard HashMap does not natively support this behavior, there are several approaches to achieve it:
1. Map with List as the Value:
This approach involves creating a HashMap where the value is a list of the desired values. For example:
Map<string list>> peopleByForename = new HashMap();</string>
While this method provides flexibility regarding the number of values stored under the key, it lacks the guarantee of having exactly two values.
2. Using a Wrapper Class:
An alternative is to define a wrapper class that contains the desired values and use it as the value in the HashMap.
class Wrapper { private Person person1; private Person person2; public Wrapper(Person person1, Person person2) { this.person1 = person1; this.person2 = person2; } public Person getPerson1() { return this.person1; } public Person getPerson2() { return this.person2; } } Map<string wrapper> peopleByForename = new HashMap();</string>
This method ensures the presence of exactly two values, but it requires the creation of additional boilerplate code for the wrapper class.
3. Using a Tuple:
A tuple class encapsulates multiple values into a single object. By using a tuple as the value in the HashMap, you can effectively achieve multiple values per key. For example:
import java.util.Tuple; Map<string tuple2 person>> peopleByForename = new HashMap();</string>
4. Multiple Maps Side-by-Side:
Finally, you can also use multiple HashMaps for different key types. For instance, you could create:
Map<string person> firstPersonByForename = new HashMap(); Map<string person> secondPersonByForename = new HashMap();</string></string>
While this method allows for clear separation of the values, it introduces the potential for inconsistency if the two maps are not consistently updated.
The choice of approach depends on the specific requirements of the application. If strict enforcement of two values per key is crucial, using a wrapper class or tuple may be more suitable. Alternatively, if flexibility in the number of values is desired, using a map with a list as the value may be more appropriate.
The above is the detailed content of How to Implement a HashMap with Multiple Values Per Key in Java?. For more information, please follow other related articles on the PHP Chinese website!
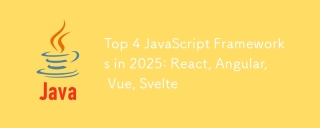
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
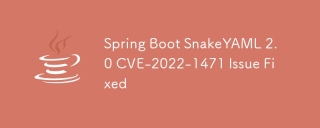
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
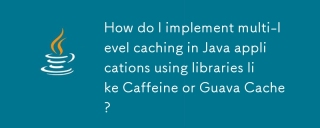
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
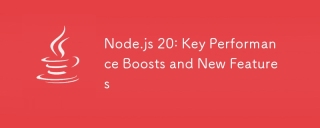
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
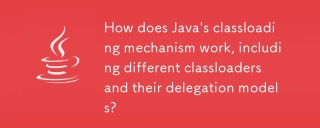
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
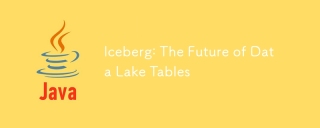
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
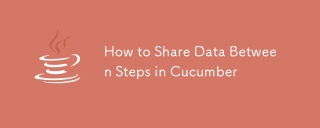
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
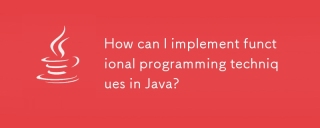
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
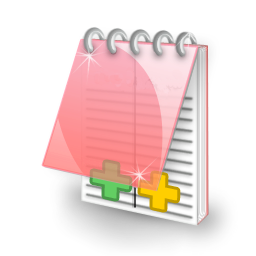
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
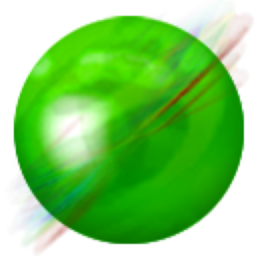
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
