


Static Polymorphism and Typedefs in C
Curiously Recurring Template Pattern (CRTP) is a technique in C to achieve static polymorphism. However, when attempting to generalize this pattern to modify return types based on derived types, the code may not compile, as encountered by a user using MSVC 2010.
Issue:
The following code, intended to allow different return types in the derived class, fails to compile:
template <typename derived_t> class base { public: typedef typename derived_t::value_type value_type; value_type foo() { return static_cast<derived_t>(this)->foo(); } }; template <typename t> class derived : public base<derived>> { public: typedef T value_type; value_type foo() { return T(); } };</derived></typename></derived_t></typename>
The error encountered is "is not a member of 'derived'.'" This occurs because the derived type is incomplete when used as a template argument for its base class.
Solution using Traits:
A common workaround is to employ a traits class template. In this approach, a base_traits class template is defined to encapsulate both types and functions from the derived class. The original base class is modified to use these traits.
template <typename derived_t> struct base_traits; template <typename derived_t> struct base { typedef typename base_traits<derived_t>::value_type value_type; value_type base_foo() { return base_traits<derived_t>::call_foo(static_cast<derived_t>(this)); } }; template <typename t> struct derived : base<derived>> { typedef typename base_traits<derived>::value_type value_type; value_type derived_foo() { return value_type(); } }; template <typename t> struct base_traits<derived>> { typedef T value_type; static value_type call_foo(derived<t>* x) { return x->derived_foo(); } };</t></derived></typename></derived></derived></typename></derived_t></derived_t></derived_t></typename></typename>
By specializing the base_traits class for each type used in the derived template argument, the desired types and functions can be accessed through the traits, enabling both static polymorphism and dynamic return types.
The above is the detailed content of How Can C Traits Solve Compilation Issues with Static Polymorphism and Typedefs in the Curiously Recurring Template Pattern?. For more information, please follow other related articles on the PHP Chinese website!
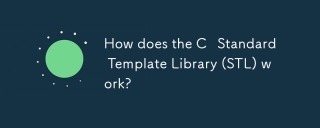
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
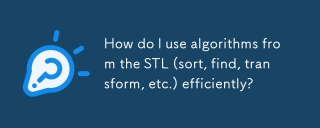
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
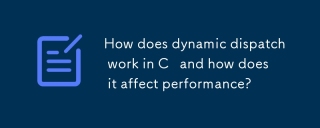
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
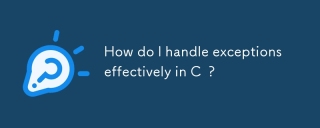
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
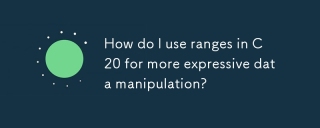
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
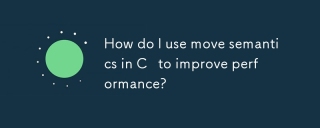
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
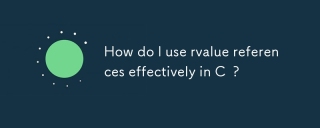
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
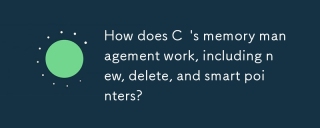
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
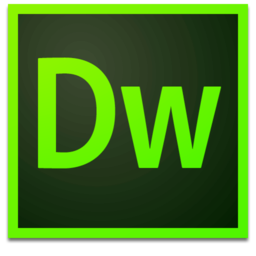
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
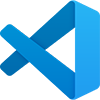
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
