


Python Functions: Delving into Return Statements
Many Python programmers face the dilemma of choosing between using return None, return, or no return statement at all when writing functions. This article explores the subtle differences among these approaches and provides guidance on when each is appropriate.
The Function Trio:
Consider the following three functions:
def my_func1(): print("Hello World") return None def my_func2(): print("Hello World") return def my_func3(): print("Hello World")
At first glance, all three functions appear to return None. However, upon closer examination, there are nuanced differences in their behavior.
Using return None:
This explicitly indicates that the function is designed to return a value, in this case None. This value can be subsequently utilized elsewhere in the code. return None is typically employed when there is a specific return value that the function is intended to provide.
For instance, the following function returns a person's mother if they are human, and None otherwise:
def get_mother(person): if is_human(person): return person.mother else: return None
Using return:
This functions similarly to break statements in loops. It primarily serves to terminate the function execution, with the return value being inconsequential. While not frequently needed, return can be useful in specific scenarios.
Consider this example, where we search for a prisoner with a knife among a group of prisoners:
def find_prisoner_with_knife(prisoners): for prisoner in prisoners: if "knife" in prisoner.items: prisoner.move_to_inquisition() return # No need to check the remaining prisoners or raise an alert raise_alert()
Note that the function's return value should not be assigned to a variable, as it is not intended for further use.
Using No return Statement:
This approach also returns None, but it implies that the function has successfully finished without any specific return value. It essentially fulfills the same role as return in void functions in languages like C or Java.
def set_mother(person, mother): if is_human(person): person.mother = mother
Conclusion:
While all three methods ultimately return None, they serve distinct purposes. return None is employed when a specific return value needs to be conveyed. return is used to explicitly terminate function execution, and using no return statement indicates a successfully completed function without a specified return value.
The above is the detailed content of Python Functions: Return None, Return, or No Return Statement—Which Should You Use?. For more information, please follow other related articles on the PHP Chinese website!
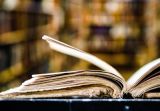
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
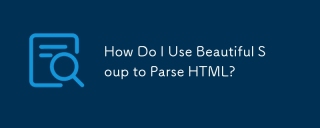
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
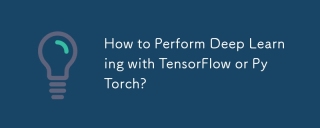
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
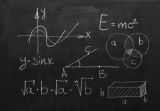
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
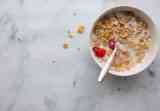
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
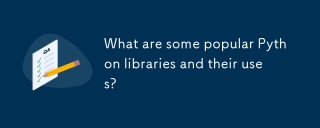
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
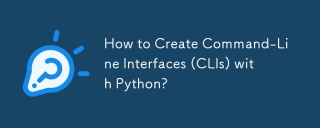
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
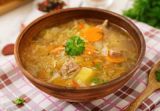
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
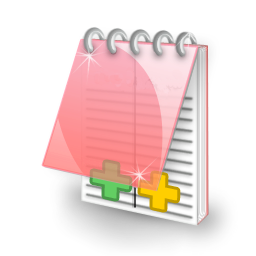
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
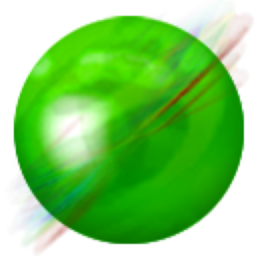
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
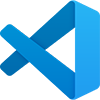
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
