


Emulating fmap Functionality in Go
In various programming languages, such as Haskell, fmap is an invaluable tool for applying functions to data structures while preserving their type. However, implementing a direct equivalent of fmap in Go presents a unique challenge due to its method-based generics. The question arises: how can we emulate fmap functionality in Go without compromising type safety?
limitations of Go Generics
Unfortunately, Go's current generics system does not lend itself well to directly emulating fmap via methods. Method parameters cannot introduce new type parameters, leading to the type mismatch error mentioned in the question.
Alternative Approaches
While it may be tempting to use a method approach similar to Haskell, the following considerations lead to a more advisable alternative:
- Using generics and methods to emulate Haskell typeclasses can lead to overly complex and potentially unexpected code.
- A more Go-like approach relies on top-level functions to achieve the desired behavior.
Implementing fmap as a Top-Level Function
The recommended way to emulate fmap in Go is to define it as a top-level function outside of any types. Here's an example:
package main import "fmt" type S[A any] struct { contents A } func Fmap[A, B any](sa S[A], f func(A) B) S[B] { return S[B]{contents: f(sa.contents)} } func main() { ss := S[string]{"foo"} f := func(s string) int { return len(s) } fmt.Println(Fmap(ss, f)) // {3} }
This approach aligns better with Go's method-based generics while preserving type safety and readability.
Conclusion
Although it may be enticing to directly translate concepts from other languages into Go, it is important to consider the inherent limitations of the Go language. By embracing a more idiomatic Go-based approach to emulating fmap, we can achieve the desired functionality while adhering to Go's design principles.
The above is the detailed content of How Can We Emulate fmap Functionality in Go While Maintaining Type Safety?. For more information, please follow other related articles on the PHP Chinese website!
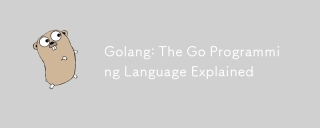
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
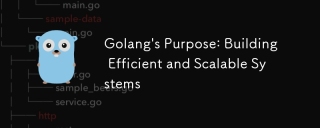
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
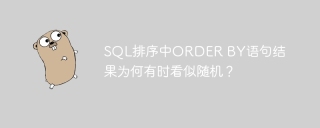
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
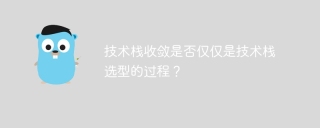
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
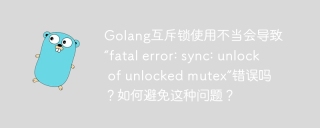
Golang ...
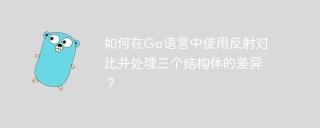
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...
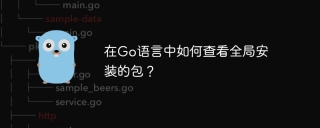
How to view globally installed packages in Go? In the process of developing with Go language, go often uses...
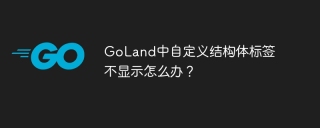
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use