How to Efficiently Sort a Vector of Pairs by Pair's Second Element
This article addresses the question of sorting a vector of pairs based on the second element of each pair in ascending order. While creating a custom function object for this task is a viable solution, there are alternative methods that utilize existing STL components and std::less.
Using std::sort with a Custom Comparator
One approach is to employ a custom comparator as an optional third argument to std::sort. This custom comparator, called sort_pred, is defined as follows:
struct sort_pred { bool operator()(const std::pair<int> &left, const std::pair<int> &right) { return left.second <p>To utilize this comparator, simply pass it to std::sort:</p> <pre class="brush:php;toolbar:false">std::sort(v.begin(), v.end(), sort_pred());
Using C 11 Lambdas
If using a C 11 compiler, you can leverage lambdas in place of a custom comparator:
std::sort(v.begin(), v.end(), [](const std::pair<int> &left, const std::pair<int> &right) { return left.second <p><strong>Using a Generic Template for Pair Sorting</strong></p> <p>For greater flexibility and reusability, you can create a generic template called sort_pair_second:</p> <pre class="brush:php;toolbar:false">template <class t1 class t2 pred="std::less<T2"> > struct sort_pair_second { bool operator()(const std::pair<t1>&left, const std::pair<t1>&right) { Pred p; return p(left.second, right.second); } };</t1></t1></class>
With this template, you can achieve the desired sorting as follows:
std::sort(v.begin(), v.end(), sort_pair_second<int int>());</int>
The above is the detailed content of How to Efficiently Sort a Vector of Pairs by the Second Element?. For more information, please follow other related articles on the PHP Chinese website!
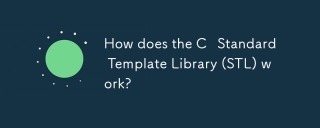
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
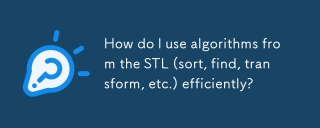
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
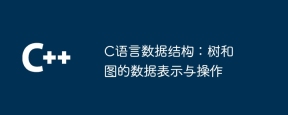
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
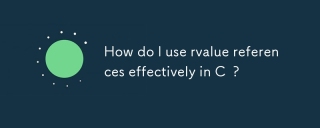
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
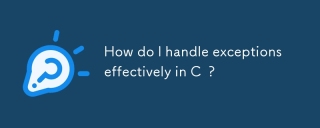
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
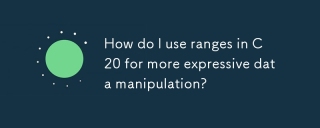
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
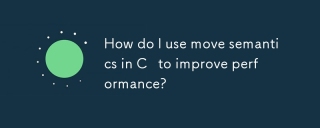
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
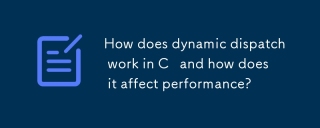
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
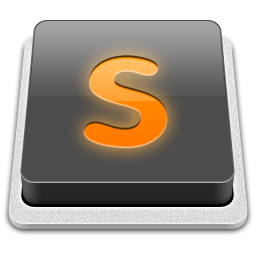
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.