How to List Files in Java Sorted by Date Modified
File management is a common task in programming, and Java provides several methods to manipulate files. When working with a directory, it is often desirable to list the files in a specific order, such as by their date of modification.
Current Solution
One approach to this is to use the File.listFiles() method to obtain an array of File objects representing the files in the directory. These files can then be sorted using the Arrays.sort() method, passing a Comparator that compares the files based on their last modified dates.
Here is an example of this approach:
File[] files = directory.listFiles(); Arrays.sort(files, new Comparator<file>() { public int compare(File f1, File f2) { return Long.valueOf(f1.lastModified()).compareTo(f2.lastModified()); } });</file>
Alternatives
This method is effective, but it may not be the most efficient approach. Sorting a large number of files can be time-consuming. A more efficient option is to use a TreeSet to maintain the files in sorted order.
Here is an example using a TreeSet:
Set<file> files = new TreeSet<file>(new Comparator<file>() { public int compare(File f1, File f2) { return Long.valueOf(f1.lastModified()).compareTo(f2.lastModified()); } }); files.addAll(directory.listFiles());</file></file></file>
The TreeSet will automatically maintain the files in sorted order based on their last modified dates. This approach is more efficient for large sets of files.
The above is the detailed content of How to Efficiently List Java Files Sorted by Modification Date?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
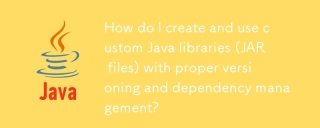
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
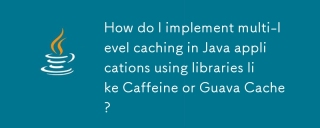
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
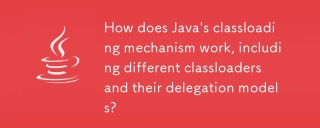
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
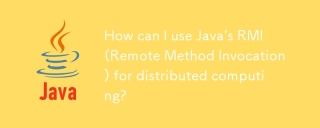
This article explains Java's Remote Method Invocation (RMI) for building distributed applications. It details interface definition, implementation, registry setup, and client-side invocation, addressing challenges like network issues and security.
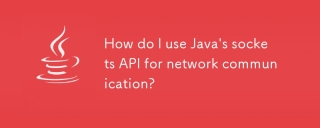
This article details Java's socket API for network communication, covering client-server setup, data handling, and crucial considerations like resource management, error handling, and security. It also explores performance optimization techniques, i
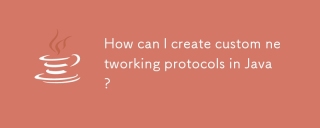
This article details creating custom Java networking protocols. It covers protocol definition (data structure, framing, error handling, versioning), implementation (using sockets), data serialization, and best practices (efficiency, security, mainta


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use