Displaying Data Streamed from a Flask View in Real Time
Introduction
When working with real-time data, it's often desirable to display the data as it becomes available. With Flask, this can be challenging as templates are rendered only once on the server side. This article explores how to overcome this limitation, allowing for the dynamic display of streamed data in a larger templated page.
Utilizing JavaScript and XMLHttpRequest
The most versatile approach involves using JavaScript and XMLHttpRequest to periodically retrieve data from a streamed endpoint. The received data can then be dynamically added to the page. This grants complete control over the output and its presentation.
# Stream endpoint that generates sqrt(i) and yields it as a string @app.route("/stream") def stream(): def generate(): for i in range(500): yield f"{math.sqrt(i)}\n" time.sleep(1) return app.response_class(generate(), mimetype="text/plain")
<!-- Utilize JavaScript to handle streaming data updates --> <script> // Retrieve latest and historical values from streamed endpoint xhr.open("GET", "{{ url_for('stream') }}"); xhr.send(); var latest = document.getElementById("latest"); var output = document.getElementById("output"); var position = 0; function handleNewData() { // Split response, retrieve new messages, and track position var messages = xhr.responseText.split("\n"); messages.slice(position, -1).forEach(function (value) { latest.textContent = value; // Update latest value var item = document.createElement("li"); item.textContent = value; output.appendChild(item); }); position = messages.length - 1; } // Periodically check for new data and stop when stream ends var timer; timer = setInterval(function () { handleNewData(); if (xhr.readyState == XMLHttpRequest.DONE) { clearInterval(timer); latest.textContent = "Done"; } }, 1000); </script>
Using an Iframe** Approach
Alternatively, an iframe can display streamed HTML output. While initially easier to implement, it introduces disadvantages such as increased resource usage and limited styling options. Nonetheless, it can be useful for certain scenarios.
# Stream endpoint that generates html output @app.route("/stream") def stream(): @stream_with_context def generate(): yield render_template_string('<link rel="stylesheet" href="%7B%7B%20url_for(" static filename="stream.css">') for i in range(500): yield render_template_string("<p>{{ i }}: {{ s }}</p>\n", i=i, s=math.sqrt(i)) sleep(1) return app.response_class(generate())
<!-- Using an iframe for displaying streamed HTML --> <p>This is all the output:</p> <iframe src="%7B%7B%20url_for(" stream></iframe>
Conclusion
Whether utilizing JavaScript or iframes, Flask allows for the integration of real-time data streaming into templated web pages. These techniques enable the dynamic display of ever-changing data, providing a more engaging and real-time user experience.
The above is the detailed content of How to Display Real-time Data Streamed from a Flask View?. For more information, please follow other related articles on the PHP Chinese website!
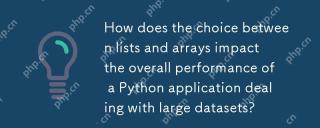
ForhandlinglargedatasetsinPython,useNumPyarraysforbetterperformance.1)NumPyarraysarememory-efficientandfasterfornumericaloperations.2)Avoidunnecessarytypeconversions.3)Leveragevectorizationforreducedtimecomplexity.4)Managememoryusagewithefficientdata
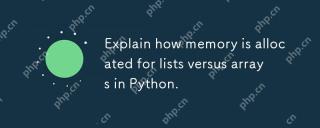
InPython,listsusedynamicmemoryallocationwithover-allocation,whileNumPyarraysallocatefixedmemory.1)Listsallocatemorememorythanneededinitially,resizingwhennecessary.2)NumPyarraysallocateexactmemoryforelements,offeringpredictableusagebutlessflexibility.
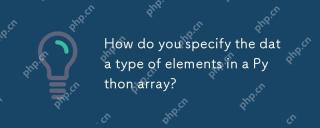
InPython, YouCansSpectHedatatYPeyFeLeMeReModelerErnSpAnT.1) UsenPyNeRnRump.1) UsenPyNeRp.DLOATP.PLOATM64, Formor PrecisconTrolatatypes.
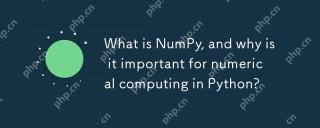
NumPyisessentialfornumericalcomputinginPythonduetoitsspeed,memoryefficiency,andcomprehensivemathematicalfunctions.1)It'sfastbecauseitperformsoperationsinC.2)NumPyarraysaremorememory-efficientthanPythonlists.3)Itoffersawiderangeofmathematicaloperation
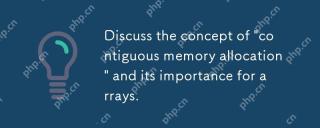
Contiguousmemoryallocationiscrucialforarraysbecauseitallowsforefficientandfastelementaccess.1)Itenablesconstanttimeaccess,O(1),duetodirectaddresscalculation.2)Itimprovescacheefficiencybyallowingmultipleelementfetchespercacheline.3)Itsimplifiesmemorym
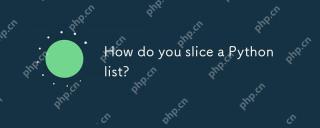
SlicingaPythonlistisdoneusingthesyntaxlist[start:stop:step].Here'showitworks:1)Startistheindexofthefirstelementtoinclude.2)Stopistheindexofthefirstelementtoexclude.3)Stepistheincrementbetweenelements.It'susefulforextractingportionsoflistsandcanuseneg

NumPyallowsforvariousoperationsonarrays:1)Basicarithmeticlikeaddition,subtraction,multiplication,anddivision;2)Advancedoperationssuchasmatrixmultiplication;3)Element-wiseoperationswithoutexplicitloops;4)Arrayindexingandslicingfordatamanipulation;5)Ag
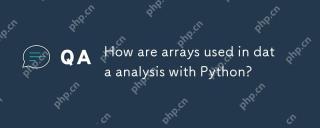
ArraysinPython,particularlythroughNumPyandPandas,areessentialfordataanalysis,offeringspeedandefficiency.1)NumPyarraysenableefficienthandlingoflargedatasetsandcomplexoperationslikemovingaverages.2)PandasextendsNumPy'scapabilitieswithDataFramesforstruc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
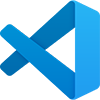
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
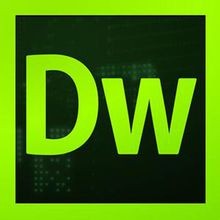
Dreamweaver CS6
Visual web development tools
