Joining Two Lists in Java Efficiently
Combining multiple lists into a single collection is a common task in Java. While the provided code segment using addAll() is functional, it involves creating a new list and iterating over both original lists, which can be inefficient for large datasets.
Java 8 and Later:
For Java 8 and subsequent versions, a more concise and efficient approach is leveraging Java streams:
List<string> newList = Stream.concat(listOne.stream(), listTwo.stream()) .collect(Collectors.toList());</string>
This code creates a new stream by concatenating the streams of both lists and collects the elements into a new list named newList. It preserves the original lists and performs the operation lazily, minimizing memory consumption and improving performance.
Java 16 and Later:
In Java 16 and later, a simplified version of the stream-based solution can be used:
List<string> newList = Stream.concat(listOne.stream(), listTwo.stream()).toList();</string>
This code utilizes the toList() method introduced in Java 16, which creates a new list directly from the stream without the need for an explicit collector.
The above is the detailed content of How Can I Efficiently Combine Two Lists in Java?. For more information, please follow other related articles on the PHP Chinese website!
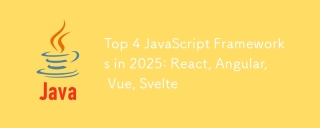
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
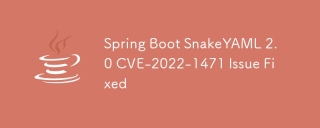
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
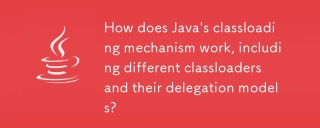
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
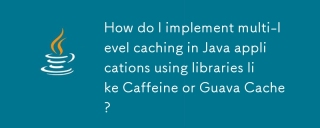
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
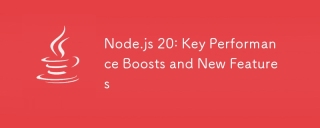
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
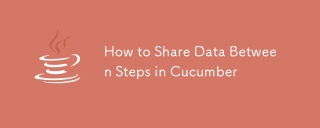
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
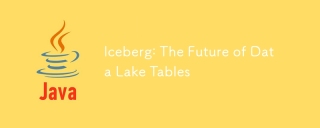
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
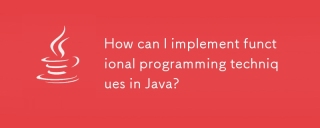
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
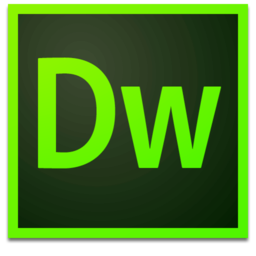
Dreamweaver Mac version
Visual web development tools
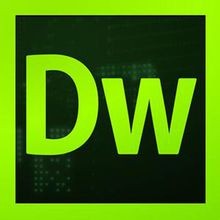
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
