Rotating Points About a Fixed Point in 2D
In order to create a realistic card-fanning effect in a card game, it is necessary to transform the coordinates of the card points to align with the rotation angle. The Allegro API provides a convenient function for rotating bitmaps, but understanding the underlying mathematical operations is crucial for collision detection purposes.
Rotation Transformation Algorithm
To rotate a point (x, y) about a fixed point (cx, cy) by an angle θ, follow these steps:
-
Subtract the Pivot Point: Subtract the x and y coordinates of the pivot point from the coordinates of the point to be rotated:
dx = x - cx dy = y - cy
-
Apply Rotation Matrix: Apply the rotation matrix to rotate the point by angle θ:
x_new = dx * cos(θ) - dy * sin(θ) y_new = dx * sin(θ) + dy * cos(θ)
-
Add the Pivot Point Back: Add the x and y coordinates of the pivot point back to the transformed coordinates:
x = x_new + cx y = y_new + cy
Implementation
Using this algorithm, here is a C-like function to perform the rotation:
POINT rotate_point(float cx, float cy, float angleInRads, POINT p) { float s = sin(angleInRads); float c = cos(angleInRads); // Translate point back to origin: p.x -= cx; p.y -= cy; // Rotate point float xnew = p.x * c - p.y * s; float ynew = p.x * s + p.y * c; // Translate point back: p.x = xnew + cx; p.y = ynew + cy; return p; }
Using this function, you can now rotate the points of the card to perform the collision detection for the mouse click events.
The above is the detailed content of How Do I Rotate a Point Around a Fixed Point in 2D?. For more information, please follow other related articles on the PHP Chinese website!
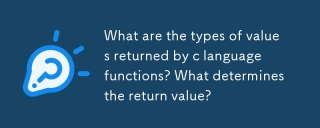
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
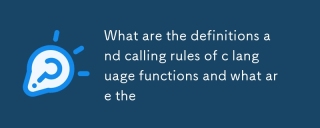
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
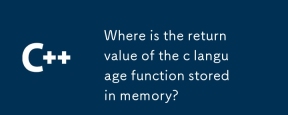
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
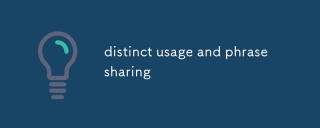
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
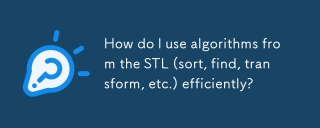
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
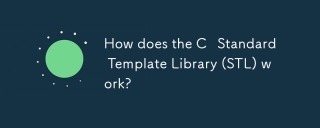
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
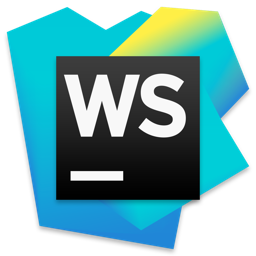
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
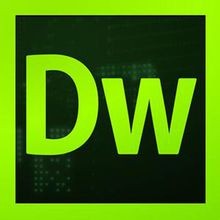
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
