


C 17 Features
C 17 introduces a wealth of new features to the language, significantly expanding its capabilities and enhancing its usability. These additions encompass various aspects of the language, from template improvements and lambda enhancements to library additions and deprecated elements.
Language Features
Templates and Generic Code:
- Template Argument Deduction for Class Templates: Constructors can now deduce template arguments, similar to functions.
-
Template Argument: Represents a value of any non-type template argument type. - Revising Non-Type Template Arguments: Several fixes and revisions have been made to non-type template arguments.
Lambda:
- Constexpr Lambdas: Lambdas can now be explicitly marked as constexpr if they satisfy certain requirements.
- Capturing this in Lambdas: Lambdas can now capture the this pointer, allowing access to member variables and methods.
Attributes:
- New Attributes: Attributes such as [[fallthrough]], [[nodiscard]], and [[maybe_unused]] have been introduced to enhance code clarity and readability.
- Attributes on Namespaces and Enumerators: Attributes can now be applied to namespaces and enumerators using [[attributes]].
Syntax Cleanup:
- Inline Variables: Variables can now be declared inline, allowing the compiler to inline their definitions.
- Simplified Namespace Declaration: The namespace A::B syntax has been introduced for concise namespace declarations.
- Simplified static_assert: static_assert statements can now be used without a string argument.
Clean Multi-Return and Flow Control:
- Structured Bindings: Structured bindings enable more convenient unpacking of tuples and other data structures in declarations.
- Conditional Statements with Initialization: if (init; condition) and switch (init; condition) provide more flexibility in conditional statements.
- Generalizing Range-Based For Loops: Range-based for loops now support sentinels (end iterators) that differ from the beginning iterators.
- if constexpr: if constexpr allows conditional compilation based on compile-time constants.
Other:
- Hexadecimal Float Point Literals: Floating-point literals can now be expressed in hexadecimal format.
- Dynamic Memory Allocation for Over-Aligned Data: Improved support for dynamic memory allocation of over-aligned data.
- Guaranteed Copy Elision: Certain scenarios where copy elision was not guaranteed now have explicit guarantees.
- Improved Order-of-Evaluation: The order-of-evaluation rules for certain expressions have been revised for clearer behavior.
Library Additions
Data Types:
- std::variant: A polymorphic type that can hold a value of one of multiple alternative types.
- std::optional: Represents an optional value that may or may not contain a value.
- std::any: A type-erased container that can hold a value of arbitrary type.
- std::string_view: A reference to a character sequence, similar to const char*.
- std::byte: A type for representing raw bytes.
Utilities:
- std::invoke: Invokes a callable with given arguments, supporting various types of callables.
- std::apply: Unpacks a tuple and passes its elements as arguments to a callable.
- std::invoke_result and Related Concepts: Concepts and types for determining the validity and result of invoking a callable with given arguments.
File System Library (TS v1):
- Classes and functions for working with file systems, directories, and files.
New Algorithms:
- for_each_n
- reduce
- transform_reduce
- exclusive_scan
- inclusive_scan
- transform_exclusive_scan
- transform_inclusive_scan
Threading:
- std::shared_mutex: A shared mutex that allows multiple readers but only one writer.
-
atomic
::is_always_lockfree: Indicates whether an atomic type is lock-free in all cases. -
scoped_lock
:: Locks multiple mutexes simultaneously, simplifying locking and unlocking.
(Partial) Library Fundamentals Library (TS v1):
- Functionality for performing searches and working with polymorphic allocators.
Container Improvements:
- try_emplace and insert_or_assign: Improved container insertion methods for handling specific scenarios.
- Splicing for Containers: Efficiently moving nodes between containers.
Smart Pointer Changes:
- Fixes and tweaks to std::unique_ptr and other smart pointers.
Additional Improvements:
- Trivially Copyable std::reference_wrapper: Improves performance in certain cases.
- Updated C11 Library: The C 17 standard library is based on C11, providing compatibility with modern systems.
- Deprecated Elements: Various elements from previous versions of the C standard have been deprecated.
Features in C 1z (Expected for C 17)**
To determine which features from the C 1z working draft will be available in C 17 compilers, it is recommended to consult the documentation and release notes of individual compilers. Not all features from C 1z will necessarily be implemented in every C 17 compiler.
Please note that not all changes or features have been mentioned in this summary. For a comprehensive list, please refer to the C 17 standard document or other reputable sources.
The above is the detailed content of What are the key language features, library additions, and deprecated elements introduced in C 17?. For more information, please follow other related articles on the PHP Chinese website!
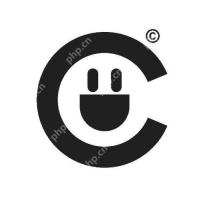
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
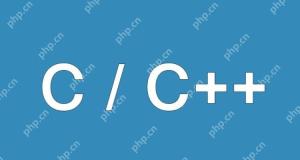
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
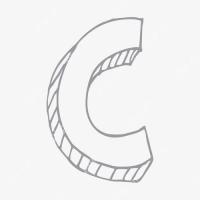
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
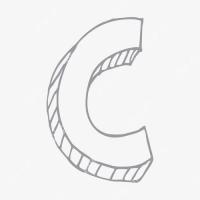
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
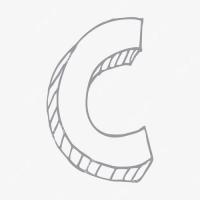
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
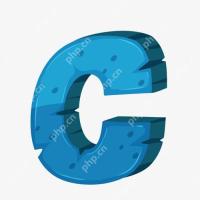
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.
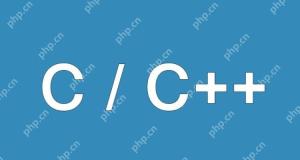
The delegate constructor in C is a function introduced by C 11, allowing one constructor to call another constructor of the same class. 1. It simplifies the writing of constructors and avoids code duplication. 2. This mechanism improves the clarity and maintainability of the code. 3. When using it, be careful to avoid loop calls, and the delegate call must be the first statement in the constructor body.
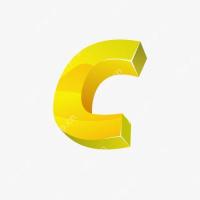
Implementing singleton pattern in C can ensure that there is only one instance of the class through static member variables and static member functions. The specific steps include: 1. Use a private constructor and delete the copy constructor and assignment operator to prevent external direct instantiation. 2. Provide a global access point through the static method getInstance to ensure that only one instance is created. 3. For thread safety, double check lock mode can be used. 4. Use smart pointers such as std::shared_ptr to avoid memory leakage. 5. For high-performance requirements, static local variables can be implemented. It should be noted that singleton pattern can lead to abuse of global state, and it is recommended to use it with caution and consider alternatives.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
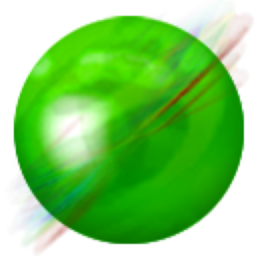
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor
