


How Does Python's Object Assignment Work, and How Can I Create Independent Copies?
Understanding Object Assignment in Python
In Python, assignment operators, such as =, do not create copies of objects. Instead, they refer to existing objects in memory. This behavior can lead to unintended consequences, as demonstrated in the following code:
dict_a = dict_b = dict_c = {} dict_c['hello'] = 'goodbye' print(dict_a) print(dict_b) print(dict_c)
Expected Output:
{} {} {'hello': 'goodbye'}
Actual Output:
{'hello': 'goodbye'} {'hello': 'goodbye'} {'hello': 'goodbye'}
In this example, we expected to create three independent dictionaries. However, the result shows that dict_a, dict_b, and dict_c all refer to the same dictionary object. Assigning a key to dict_c modifies the shared dictionary, which is reflected in all three variables.
Resolving Object Assignment Issues
To achieve the desired behavior of creating independent copies, Python provides several options:
- dict.copy() method: This method creates a shallow copy of the dictionary, copying all its key-value pairs into a new object.
dict_a = {1: 2} dict_b = dict_a.copy() dict_b[3] = 4 print(dict_a) print(dict_b)
Output:
{1: 2} {1: 2, 3: 4}
- copy.deepcopy() function: This function creates a deep copy of the dictionary, including all nested objects.
import copy dict_a = {1: 2, 'nested': {3: 4}} dict_b = copy.deepcopy(dict_a) dict_b['nested'][5] = 6 print(dict_a) print(dict_b)
Output:
{1: 2, 'nested': {3: 4}} {1: 2, 'nested': {3: 4, 5: 6}}
By using these methods, it is possible to control object assignment in Python and prevent unintended modifications to shared objects.
The above is the detailed content of How Does Python's Object Assignment Work, and How Can I Create Independent Copies?. For more information, please follow other related articles on the PHP Chinese website!
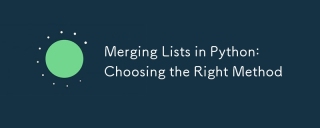
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
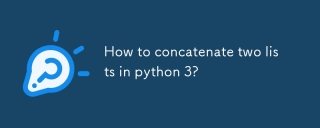
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
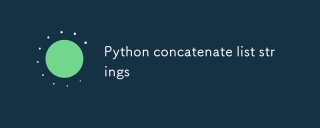
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
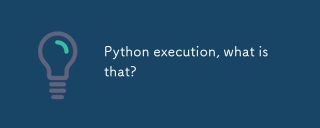
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
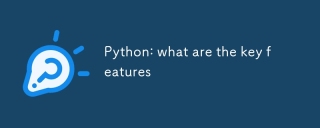
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
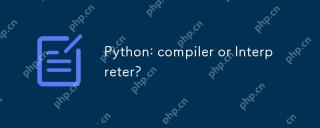
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
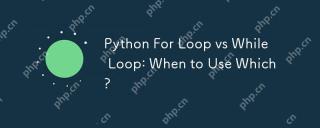
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
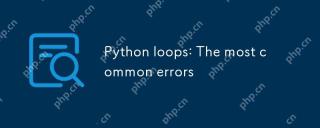
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
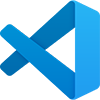
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
