Gson Field Exclusion Without Annotations
In Gson, you can exclude specific fields from serialization without using annotations. Let's explore an alternative approach using the GsonBuilder.setExclusionStrategies() method.
The ExclusionStrategy interface allows you to control which fields are excluded or included during serialization. However, the given FieldAttributes information may not be sufficient to pinpoint specific field paths.
To address this issue, consider extending the ExclusionStrategy interface and defining your own exclusion criteria. You can use regular expressions to match specific field paths and exclude them accordingly. This approach provides greater flexibility in specifying the fields to be excluded.
Here's an example of how you can achieve field exclusion based on a field path:
import com.google.gson.ExclusionStrategy; import com.google.gson.FieldAttributes; public class CustomExclusionStrategy implements ExclusionStrategy { private String[] excludedPaths; public CustomExclusionStrategy(String[] excludedPaths) { this.excludedPaths = excludedPaths; } @Override public boolean shouldSkipField(FieldAttributes fieldAttributes) { for (String excludedPath : excludedPaths) { if (fieldAttributes.getDeclaringClass().getName() + "." + fieldAttributes.getName().equals(excludedPath)) { return true; } } return false; } @Override public boolean shouldSkipClass(Class> clazz) { return false; } }
You can then use this custom exclusion strategy in your GsonBuilder:
Gson gson = new GsonBuilder() .setExclusionStrategies(new CustomExclusionStrategy("country.name")) .create();
This approach allows you to exclude specific field paths, such as "country.name", during serialization. You can extend this strategy to support more complex exclusion criteria as needed.
The above is the detailed content of How to Exclude Gson Fields Without Annotations?. For more information, please follow other related articles on the PHP Chinese website!
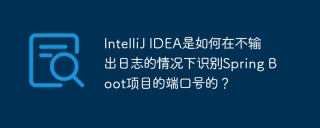
Start Spring using IntelliJIDEAUltimate version...
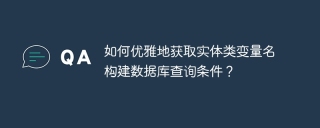
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
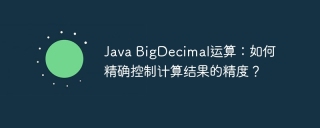
Java...
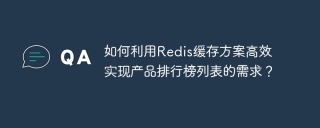
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
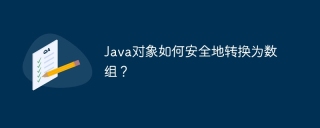
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
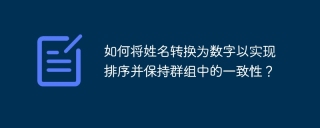
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
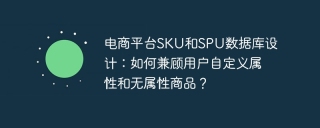
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
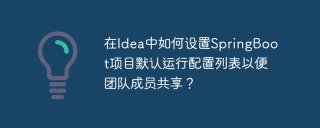
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
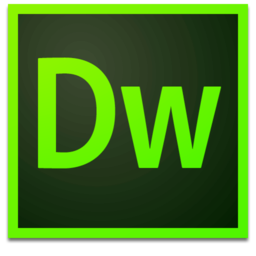
Dreamweaver Mac version
Visual web development tools
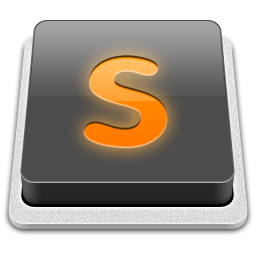
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
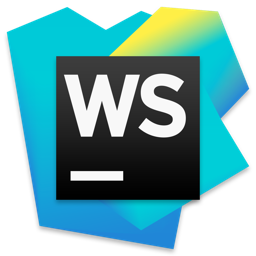
WebStorm Mac version
Useful JavaScript development tools