E2E Tests with Cypress teaches you how to perform end-to-end (E2E) testing using Cypress, one of the most popular tools for automated testing in JavaScript, especially for web applications. I will explain all the concepts and steps in detail.
What are E2E Tests?
End-to-End Tests (E2E) are automated tests that verify the complete behavior of an application, from start to finish, simulating user interaction with the interface. These tests are important because they validate that all parts of the application work correctly together, as expected, in a real environment.
Cypress: What is it and How Does It Work?
Cypress is a tool for automated testing of web applications. It is designed to be easy to use, powerful and fast. It allows you to write tests that interact with the application's user interface in the same way a user would, clicking buttons, filling out forms, validating texts, and much more.
Some important features of Cypress:
- Real-time tests: It runs the tests in the browser itself, allowing you to see the tests running live.
- Ease of setup: No complicated setup required to get started.
- Fast execution: Since Cypress runs in the browser, test execution is faster compared to other E2E testing tools.
- CI/CD Integration: It easily integrates with CI/CD pipelines for test automation.
Module 34 Walkthrough
1. Conceptualizing the Cypress tool
Cypress is an automated testing tool for web applications, mainly for E2E testing. It is designed to interact directly with application code in the browser, making testing more efficient.
2. Installing Cypress on the machine
To start using Cypress, you need to install it in your project. Here is the installation command:
npm install cypress --save-dev
This will install Cypress as a development dependency in your project.
3. Starting Cypress
After installing Cypress, you can open it using the following command in the terminal:
npm install cypress --save-dev
This will open the Cypress Test Runner where you can see the tests running in the browser. It also creates a cypress folder in your project, where all tests and configurations are stored.
4. Using the describe function to group tests
In Cypress (and Jest), we use describe to group multiple tests that are part of the same suite or module. This helps to organize the tests in a more structured way.
npx cypress open
In the example above:
- describe is used to group login related tests.
- it defines a specific test within the group. What follows inside it is the code that performs the verification (test steps).
5. Using the it function
The it function is used to define individual test cases. Each test case must be independent and represent a specific functionality or behavior of the application.
6. Retrieving elements with the cy.get function
The cy.get function is used to select page elements to interact with in tests.
Example:
describe('Teste de Login', () => { it('Deve realizar o login com sucesso', () => { cy.visit('https://exemplo.com/login'); cy.get('input[name="username"]').type('usuario'); cy.get('input[name="password"]').type('senha123'); cy.get('button[type="submit"]').click(); cy.url().should('include', '/dashboard'); }); });
Here, cy.get searches for the input with name="username" and the submit button with type="submit", and then performs the actions of type and click.
7. Introduction to VScode and Autocomplete
You can use VSCode to edit your tests and take advantage of Cypress autocomplete, which makes it easy to write tests correctly by suggesting methods and commands as you type.
8. Using Hierarchical Relationships
Cypress allows you to select elements based on the page hierarchy, using more complex CSS selectors. For example, you can select a button that is inside a div with a specific class:
cy.get('input[name="username"]').type('usuario'); cy.get('button[type="submit"]').click();
9. Building Filtering Tests
An example of a filtering test would be to check whether, when applying a filter, the list of items is updated correctly. Cypress allows you to perform this type of test easily, interacting with the filters and checking the results.
cy.get('.modal').find('button').click(); // Encontra o botão dentro de .modal e clica
10. Using the beforeEach function
The beforeEach function is useful for configuring the application state before each test. This is especially important when you need to ensure that the application is in an initial state before running the test.
npm install cypress --save-dev
11. Knowing Cypress Return Type
Cypress uses Promises to manage asynchronous time, but it automatically handles these promises, making testing simpler. It is not necessary to use await or .then() in many cases as Cypress handles this internally.
12. Organizing the Code to Reduce Lines
Keeping tests organized and reusable is essential. You can create helper functions and reuse code snippets.
Example:
npx cypress open
13. Knowing Additional Features of Cypress
- Take Screenshots: Cypress can automatically take screenshots during testing. This helps you visualize what happened when a test failed.
describe('Teste de Login', () => { it('Deve realizar o login com sucesso', () => { cy.visit('https://exemplo.com/login'); cy.get('input[name="username"]').type('usuario'); cy.get('input[name="password"]').type('senha123'); cy.get('button[type="submit"]').click(); cy.url().should('include', '/dashboard'); }); });
Generate Reports: Cypress allows you to generate test execution reports, which makes it easier to analyze the results.
Cypress Run: To run the tests in headless mode (without a graphical interface), use the command:
cy.get('input[name="username"]').type('usuario'); cy.get('button[type="submit"]').click();
- HTTP Request Mocking: You can simulate server responses with Cypress, without having to make real calls. This is useful for testing scenarios with specific data.
cy.get('.modal').find('button').click(); // Encontra o botão dentro de .modal e clica
14. Mock Service Worker (MSW) Installation
Mock Service Worker is a tool that allows you to intercept HTTP requests in your tests. It can be used with Cypress to simulate requests and control responses.
cy.get('.filter').select('Option 1'); cy.get('.item-list').should('have.length', 5);
Then you can configure network handlers to intercept the requests.
Conclusion
In Module 34, you learned how to use Cypress to perform E2E tests on your application, ensuring that it works correctly in real use situations. You learned how to configure Cypress, write tests, interact with page elements, and use features like beforeEach, cy.get, screenshots, reports, and much more. These tests are crucial to ensure that your application works correctly and that new bugs are not introduced.
The above is the detailed content of Ecom Cypress Tests. For more information, please follow other related articles on the PHP Chinese website!
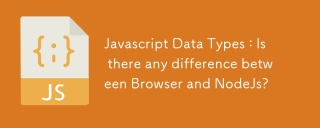
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
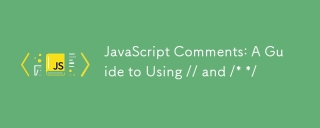
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
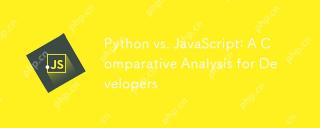
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
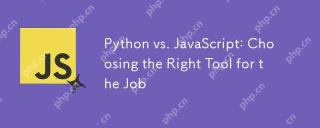
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
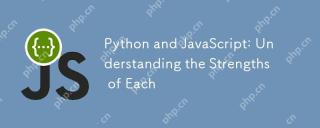
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
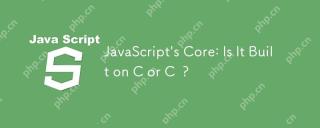
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
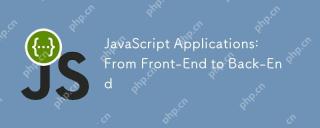
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
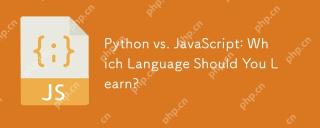
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
