How to Manage jQuery Plugin Dependencies in Webpack
When using webpack, it's common to organize application code and libraries into separate bundles. For instance, one might create a "bundle.js" for all custom code and a "vendors.js" for libraries such as jQuery and React. However, challenges arise when incorporating plugins that depend on jQuery and are desired within "vendors.js."
ProvidePlugin: Global Variable Injection
One approach is to utilize the ProvidePlugin, which injects implicit globals when encountering specific identifiers, such as "$" or "jQuery." By configuring webpack, one can instruct it to prepend "var $" when encountering "$" globally.
Example:
plugins: [ new webpack.ProvidePlugin({ $: "jquery", jQuery: "jquery" }) ]
imports-loader: This Binding Configuration
The imports-loader allows manual variable injection. Some legacy modules assume the presence of "this" as the window object, which can conflict with CommonJS contexts where "this" equates to "module.exports." The imports-loader can override this behavior and bind "this" to the window object.
Example:
module: { loaders: [ { test: /[\/\]node_modules[\/\]some-module[\/\]index\.js$/, loader: "imports-loader?this=>window" } ] }
imports-loader: AMD Disabling
Certain modules support multiple module styles, including AMD and CommonJS. However, they may prioritize define and employ unconventional exporting methods. This can be circumvented by forcing CommonJS by setting "define = false" with the imports-loader.
Example:
module: { loaders: [ { test: /[\/\]node_modules[\/\]some-module[\/\]index\.js$/, loader: "imports-loader?define=>false" } ] }
script-loader: Global Script Importation
If global variables are not a concern and simply executing legacy scripts is the objective, the script-loader can be used. It runs modules within a global context, akin to using a <script> tag.</script>
noParse: Unparsed Module Inclusion
When a module lacks AMD/CommonJS versions and a dist is desired, it can be flagged as "noParse." This instructs webpack to include the module without parsing, thereby improving build times. However, this renders features like ProvidePlugin inaccessible.
Example:
module: { noParse: [ /[\/\]node_modules[\/\]angular[\/\]angular\.js$/ ] }
By leveraging these approaches, you can effectively manage jQuery plugin dependencies within your webpack configuration.
The above is the detailed content of How to Effectively Manage jQuery Plugin Dependencies with Webpack?. For more information, please follow other related articles on the PHP Chinese website!
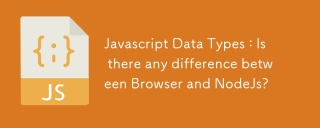
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
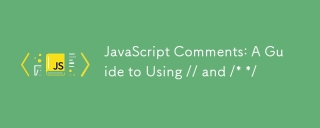
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
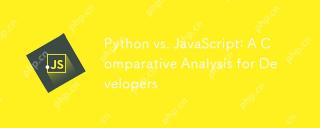
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
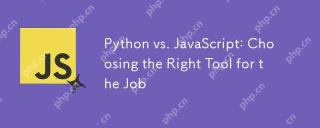
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
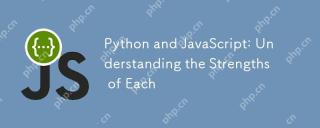
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
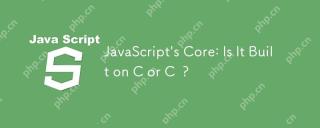
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
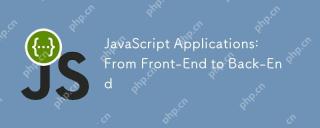
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
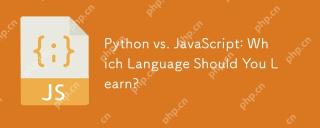
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
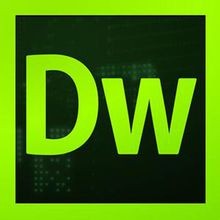
Dreamweaver CS6
Visual web development tools
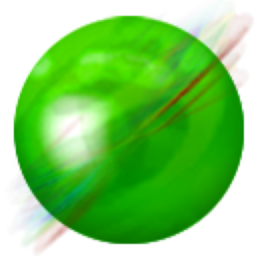
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
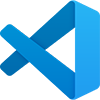
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
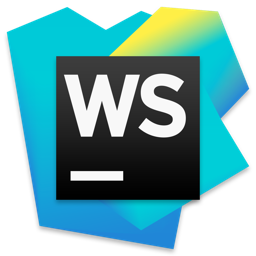
WebStorm Mac version
Useful JavaScript development tools
