Introduction
In the realm of web development, JavaScript remains a cornerstone technology. While frameworks and libraries can simplify development, mastering Vanilla JavaScript provides a solid foundation for understanding the language's intricacies. As a best practice, I recommend that developers, especially those new to the field, focus on honing their Vanilla JavaScript skills before exploring frameworks.
The Importance of Tracking User Login Status
Effectively managing user login status is crucial for ensuring the security, efficiency, and overall user experience of web applications. The benefits of implementing user login tracking include:
- FIRST PART
- Enhanced Security: Prevent unauthorized access to sensitive data by requiring users to log in before gaining access. Optimized Resource Utilization: Minimize unnecessary requests to the server by storing data locally and updating only when changes occur.
Data Integrity: Ensure data consistency by controlling modifications, additions, and deletions.
Improved User Experience: Utilize timestamps to automatically log out inactive users, enhancing security and streamlining the user experience.Seamless Server Interaction: Establish a robust communication channel between the client and server, enabling efficient data exchange and synchronization.
By implementing a well-designed user login tracking system, developers can significantly enhance the security, performance, and overall quality of their web applications.
- SECOND PART:
now Lets us dive into the code:
firstly, lets a variable to access device local storage:
const storage = window.localStorage;
secondnly we gonna create a variable that has the value of initial/default data.
The same data values will be updated whenever new data arrived or changed.
here is the variable:
const initialState = { userData: storage.getItem('exampleUserData') || null, timestamp: null, isLoggedIn: false };
- THIRD PART:
now lets create a function to save data into a device local storage:
function cacheUserData(data) { storage.setItem('exampleUserData', JSON.stringify(data)); }
now lets create our main part of the code,
which is our reducer funtion,
this function will be responsible for controlling data by insert, update, delete from our device local storage.
Here is the code:
function myReducer(state = initialState, action) { switch(action.type) { case "LOGIN": cacheUserData(action.payload); return { userData: action.payload, timestamp: Date.now(), isLoggedIn: true }; case "LOGOUT" : storage.removeItem('exampleUserData'); return { userData: null, timestamp: null, isLoggedIn: false }; default: return state; } };
Let's break down this code step by step:
Function Signature
JavaScript
function myReducer(state = initialState, action) { // ... }
This is a reducer function, which is a key concept in state management libraries like Redux. The reducer takes two arguments:
state: The current state of the application. If no state is provided, it defaults to initialState. action: An object that describes the action to be performed.
Switch Statement
JavaScript
switch (action.type) { // ... }
This switch statement checks the type property of the action object and executes the corresponding code block.
LOGIN Case
JavaScript
const initialState = { userData: storage.getItem('exampleUserData') || null, timestamp: null, isLoggedIn: false };
When the action.type is "LOGIN", the reducer:
function cacheUserData(data) { storage.setItem('exampleUserData', JSON.stringify(data)); }
LOGOUT Case
JavaScript
function myReducer(state = initialState, action) { switch(action.type) { case "LOGIN": cacheUserData(action.payload); return { userData: action.payload, timestamp: Date.now(), isLoggedIn: true }; case "LOGOUT" : storage.removeItem('exampleUserData'); return { userData: null, timestamp: null, isLoggedIn: false }; default: return state; } };
When the action.type is "LOGOUT", the reducer:
function myReducer(state = initialState, action) { // ... }
Default Case
JavaScript
default:
return state;
If the action.type doesn't match any of the above cases, the reducer simply returns the current state without making any changes.
In summary, this reducer function manages the user login state by responding to "LOGIN" and "LOGOUT" actions.
Finally but not least, the following is the function that will be used as an output of the correct data.
Important: We were suppoesd to add export to this function so that it can be use in other files, but because here it is in a single file, we dont have to. You can visit the github link bellow, to see a bigger project that has the same functionality.
User Data Management Functionality
In this section, we'll explore the userData.js function, which plays a crucial role in managing user data.
userData Function Code
JavaScript:
state: The current state of the application. If no state is provided, it defaults to initialState. action: An object that describes the action to be performed.
Code Breakdown
Let's dissect the userData function step by step:
Function Signature
JavaScript
switch (action.type) { // ... }
This asynchronous function, userData, accepts two parameters:
case "LOGIN": cacheUserData(action.payload); return { userData: action.payload, timestamp: Date.now(), isLoggedIn: true };
Fetching User Data
JavaScript
Calls the cacheUserData function with the action.payload (which contains the user data). Returns a new state object with the following properties: userData: The user data from the action.payload. timestamp: The current timestamp. isLoggedIn: Set to true.
This line fetches user data from the specified urlLink using the fetchUserData function. The await keyword ensures that the code waits for the promise to resolve before proceeding.
Calling the Reducer
JavaScript:
case "LOGOUT": storage.removeItem('exampleUserData'); return { userData: null, timestamp: null, isLoggedIn: false };
This line calls the myReducer function, passing:
Removes/dete the user data from storage using storage.removeItem. Returns a new state object with the following properties: userData: Set to null. timestamp: Set to null. isLoggedIn: Set to false.
The reducer returns a new state object, which is assigned to the state variable.
Updating State Properties
JavaScript:
const userData = async (type) => { const userData = await myUserData; const state = myReducer(undefined, { type: type, payload: userData }); state.timeStamp = state.timestamp; state.isLoggedIn = state.isLoggedIn; return state; };
These lines update two properties of the state object:
const userData = async (type) => { // ... }
Returning the State
JavaScript
return state;
Finally, the function returns the updated state object.
Example Output
When we call the userData function with different actions, we get the following output:
Login Data:
JSON
urlLink: A URL link used to fetch user data. type: A string indicating the type of action (e.g., "LOGIN" or "LOGOUT").
Logout Data:
JSON
const userData = await fetchUserData();
As you can see, our code is capable of removing data when the user logs out. We can also utilize the timeStamp to automatically log out the user after a prolonged period of inactivity or when the website tab is closed.
For a full code including dom manupulation visit the github link:
https://github.com/TrevoDng/logig-status-monitor-frontend
The above is the detailed content of Vanilla JavaScript Login Status Monitor. For more information, please follow other related articles on the PHP Chinese website!
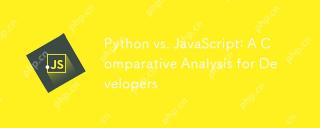
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
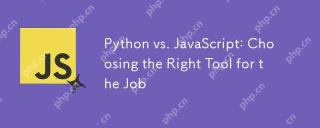
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
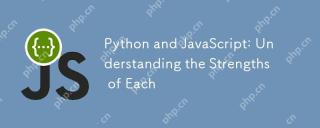
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
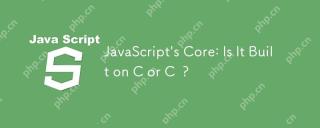
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
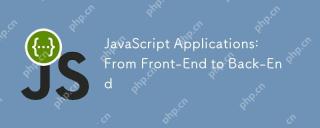
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
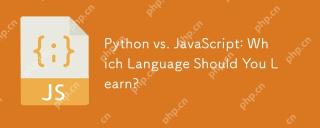
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
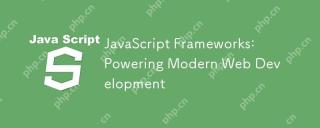
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
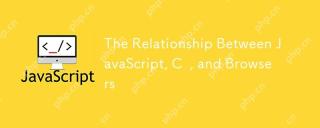
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
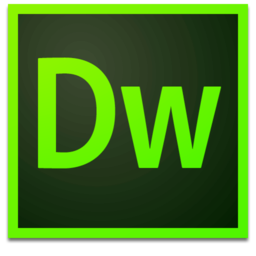
Dreamweaver Mac version
Visual web development tools
