


How Can I Safely Truncate Multibyte Strings in PHP While Preserving Word Boundaries?
Truncating Multibyte Strings in PHP
In PHP, truncating multibyte strings can be a complex task. This article addresses the challenge of truncating such strings to a specified number of characters, considering both multibyte character encoding and word boundaries.
To achieve this, one approach involves using PHP's built-in mb_strimwidth() function, which allows for the truncation of strings with specified widths. However, this function does not take into account word boundaries.
Custom Implementation for Truncation
A custom implementation can be created to handle both multibyte character encoding and word boundaries:
- Calculate Truncation Length: Subtract the length of the terminator string from the maximum number of characters to truncate.
- Validate String Length: Check if the input string is longer than the calculated truncation length; otherwise, return it unaltered.
- Find Word Boundary: Use mb_strrpos() to search for the last space character in the string below the truncation length.
- Cut String: If a word boundary is found, truncate the string at that point; otherwise, truncate at the calculated truncation length.
- Append Terminator: Add the terminator string to the truncated string.
- Return Truncated String: Output the truncated and appended string.
Example Usage:
function truncate($string, $chars = 50, $terminator = ' …') { // Calculate truncation length $trunc_len = $chars - strlen($terminator); // Validate string length if (strlen($string) <p>This function can be used to truncate multibyte strings, considering both character encoding and word boundaries. It provides a straightforward and robust solution for this common PHP task.</p>
The above is the detailed content of How Can I Safely Truncate Multibyte Strings in PHP While Preserving Word Boundaries?. For more information, please follow other related articles on the PHP Chinese website!
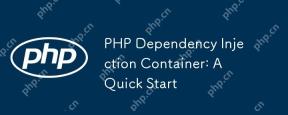
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
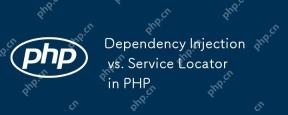
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
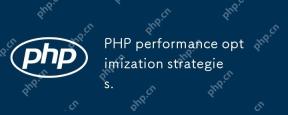
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
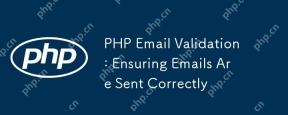
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
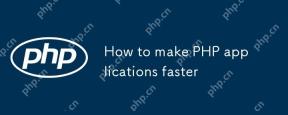
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
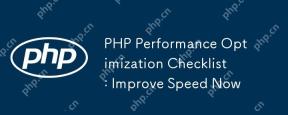
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
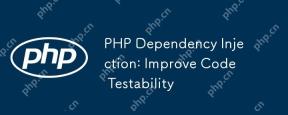
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
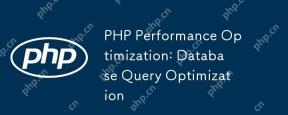
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
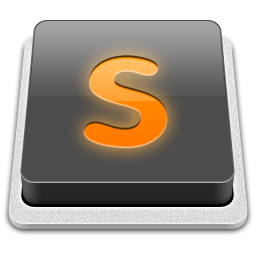
SublimeText3 Mac version
God-level code editing software (SublimeText3)
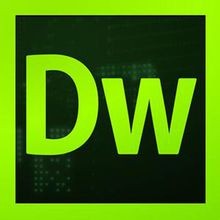
Dreamweaver CS6
Visual web development tools
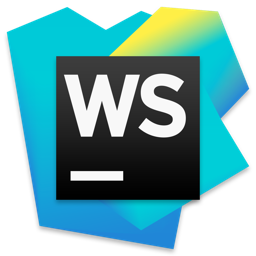
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
