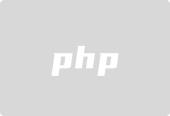
Creating Text Input Boxes in Pygame
In Pygame, you can create text input boxes to gather user input. Here's a detailed guide:
Defining the Input Box Area
Start by defining a rectangular area that represents the input box. This area will constrain the user's input.
input_box = pg.Rect(x, y, width, height)
Handling User Interaction
To detect user input, you'll need to handle relevant events:
-
MOUSEBUTTONDOWN: Check if the click occurred within the input box area.
-
KEYDOWN: Capture key presses while the input box is active.
Storing and Displaying Text
Once input is captured, you need to store it in a string and display it within the input box.
-
active = False: Initialize a flag to keep track of the input box's active state.
-
text = '' : Initialize the input text as an empty string.
-
txt_surface = FONT.render(text, True, color) : Render the text using a specified font, color, and active state.
Drawing the Input Box
Draw the input box and its corresponding text:
-
screen.blit(txt_surface, (input_box.x 5, input_box.y 5)) : Blit the text within the input box area.
-
pg.draw.rect(screen, color, input_box, 2) : Draw a rectangle around the input box.
Additional Features
-
Resizing the Input Box: If the entered text exceeds the initial box width, you can dynamically resize the box to accommodate the user's input.
-
Object-Oriented Approach: For greater flexibility, you can create InputBox classes to instantiate multiple input boxes with varying sizes and positions.
-
Third-Party Modules: Libraries like pygame_textinput offer expanded functionality for text input handling.
The above is the detailed content of How Can I Create and Manage Text Input Boxes in Pygame?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn