


How to Replace Pandas' Deprecated `append` Method for Adding Rows to a DataFrame?
Error "DataFrame' object has no attribute 'append'": Using 'concat' Instead
In pandas, the 'append' method has been removed as of version 2.0 and replaced by 'concat'. This means you can no longer use DataFrame.append() to add a dictionary as a new row to a DataFrame. To resolve this error and achieve the desired functionality, you should use 'concat' instead.
Using 'concat'
To append a dictionary as a new row to a DataFrame using 'concat', follow these steps:
-
Convert the dictionary to a DataFrame with one row:
new_row_df = pd.DataFrame([new_row])
-
Concatenate the new row DataFrame with the original DataFrame:
df = pd.concat([df, new_row_df], ignore_index=True)
Alternative: Using 'loc' (with Caution)
Another option, but with some restrictions, is to use 'loc':
df.loc[len(df)] = new_row
However, note that this only works if the new index is not already present in the DataFrame (typically the case if the index is a RangeIndex).
Why Was 'append' Removed?
The 'append' method was removed because it was inefficient for repeated insertion. While 'list.append' is O(1) at each step, 'DataFrame.append' was O(n), making it slow for loops. Additionally, it created a new DataFrame for each step, leading to a quadratic behavior.
Best Practices for Repeated Insertion
If you need to repeatedly append rows to a DataFrame, it's best to collect the new items in a list, convert it to a DataFrame, and then concatenate it to the original DataFrame at the end of the loop. This approach avoids the overhead of repeated append operations.
The above is the detailed content of How to Replace Pandas' Deprecated `append` Method for Adding Rows to a DataFrame?. For more information, please follow other related articles on the PHP Chinese website!
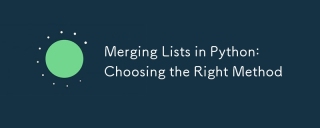
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
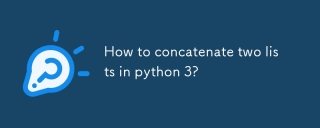
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
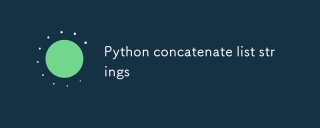
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
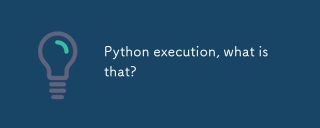
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
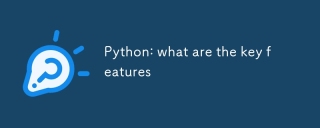
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
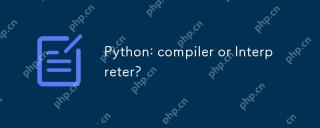
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
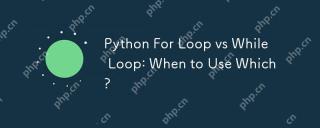
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
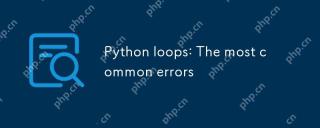
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
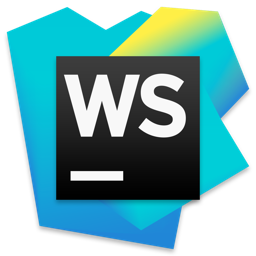
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
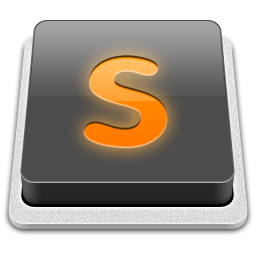
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
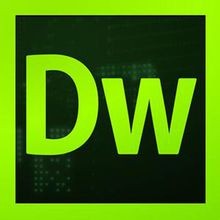
Dreamweaver CS6
Visual web development tools
