Lambda Function Optimization
Nicolai Josuttis claims in "The C Standard Library" that compilers optimize lambdas better than plain functions. This raises the question of why this is the case.
Inline Optimization
One might assume that inline optimization wouldn't differentiate between lambdas and plain functions. However, the key difference lies in the nature of lambdas as function objects.
Function Objects vs. Function Pointers
When a lambda is passed to a function template, it creates a new function specifically for that object, resulting in a trivially inlinable function call. In contrast, plain functions pass function pointers, which typically cause problems for inline optimization. Compilers can theoretically inline such calls, but only if the surrounding function is also inlined.
Example
Consider a function template map that takes an iterator and a function object as parameters:
template <typename iter typename f> void map(Iter begin, Iter end, F f) { for (; begin != end; ++begin) *begin = f(*begin); }</typename>
Invoking this template with a lambda:
int a[] = { 1, 2, 3, 4 }; map(begin(a), end(a), [](int n) { return n * 2; });
Creates a new instantiation of the function:
template void map<int _some_lambda_type>(int* begin, int* end, _some_lambda_type f) { for (; begin != end; ++begin) *begin = f.operator()(*begin); }</int>
The compiler can readily inline calls to the lambda's operator().
However, when using a function pointer:
int a[] = { 1, 2, 3, 4 }; map(begin(a), end(a), &my_function);
The resulting instantiation becomes:
template void map<int int>(int* begin, int* end, int (*f)(int)) { for (; begin != end; ++begin) *begin = f(*begin); }</int>
Here, f points to a different address for each call to map, prohibiting inline optimization unless the surrounding call to map is also inlined.
Thus, the optimization advantage of lambdas stems from their ability to create function objects that enable trivial inline capabilities.
The above is the detailed content of Why Do Compilers Optimize Lambdas Better Than Plain Functions?. For more information, please follow other related articles on the PHP Chinese website!

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
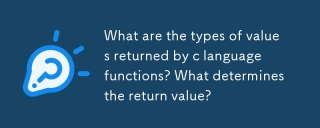
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing
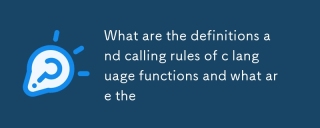
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
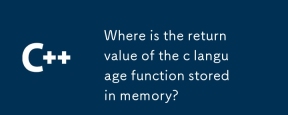
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
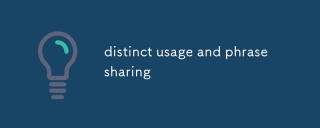
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
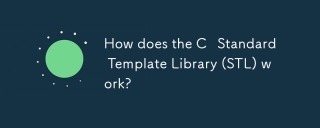
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
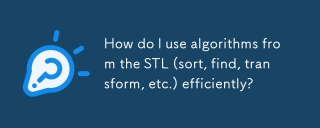
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
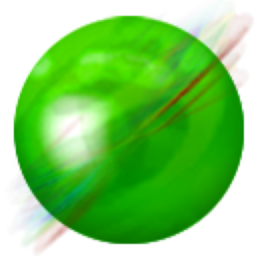
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
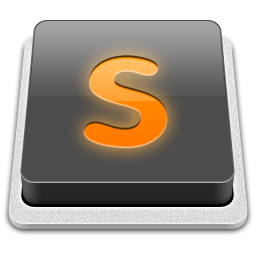
SublimeText3 Mac version
God-level code editing software (SublimeText3)
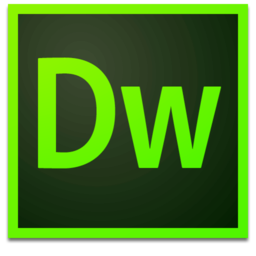
Dreamweaver Mac version
Visual web development tools
