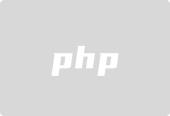
One of the most common things (or should be) when we are developing a project is to ask ourselves, what are the good programming practices that we should use. Following this context, I created a compilation of good practices that I usually follow both in my personal projects and in the professional environment.
In the next few lines, I will explore 7 good practices and development patterns that I follow and help me write better codes, improving not only quality, but also my productivity and that of the team I'm part of.
1. Adopt Consistent Naming Conventions
Consistency is one of the keys to keeping code organized and easy to understand. Following naming conventions helps ensure that everyone on the team follows a clear standard, avoiding confusion. In Java, the most commonly used conventions include:
-
Classes and Interfaces: use PascalCase (example: User, Car).
-
Methods and Variables: the most appropriate convention here is camelCase (example: calculateTotal, customerName).
-
Constants: for constants, use capital letters and separation by underscore (example: MAXIMUM_SIZE, DEFAULT_VALUE).
-
Packages: package names must be written in lowercase letters and follow the reverse domain name pattern, that is, the name of the domain inverted.
These patterns make the code easier to read and understandable for any developer working on the project.
2. Apply Object Oriented Programming (OOP)
Object-oriented programming is one of the fundamental principles of Java, and adopting its appropriate practices can make a big difference in the design of your code. OOP allows for better organization, code reuse and ease of maintenance. Some guidelines include:
-
Encapsulation: protect data within your classes and allow access to this data only through controlled methods (GETs and SETs). Using modifiers such as private or protected can prevent unwanted access.
-
Inheritance: although useful, inheritance must be used with caution so as not to generate complex and difficult-to-manage hierarchies. Prefer composition when possible.
-
Polymorphism: makes it easier to swap object behaviors at runtime, which can be useful for making your code more flexible.
Following these principles helps create more modular code, which makes changes and expansion easier in the future.
3. Avoid Redundancy with the DRY (Don't Repeat Yourself) Principle
Code duplication can lead to errors and maintenance difficulties. The DRY principle suggests that you should avoid writing the same piece of code multiple times. When you find a repeating pattern, refactor it into a reusable function or class. This makes maintenance easier and reduces the risk of bugs.
For example, if you have data validation code that repeats across multiple parts of your program, consider extracting that code into a single method or even a specific class. This improves clarity and makes the code easier to refactor in the future.
4. Comments: When to Use and When to Avoid
Comments are a powerful tool for explaining the logic behind difficult code decisions, but they should not be used to explain the obvious. If the code is well written and follows good naming practices, it should be self-explanatory. Use comments to:
- Describe complex design decisions.
- Explain why an approach was chosen when there is no clear solution.
- Provide additional context in cases involving complicated logic or specific requirements.
Comments are important to explain the "why" of a code decision, but not the "what" or "how". -Blog: Cubos Academy
Avoid comments simply to "explain" what a line of code does — good code should be able to speak for itself.
5. Automated Testing: Ensuring Code Quality
One of the best ways to ensure your code works correctly is to write automated tests. They help identify problems early, prevent regressions, and improve implementation confidence. The main types of tests include:
-
Unit Tests: test isolated units of code, such as methods or functions. Using tools like JUnit can automate this process.
-
Integration Tests: evaluate the interaction between different parts of the system. They are crucial to ensuring that the system as a whole functions as expected.
Adding automated tests to your workflow may seem laborious at first, but in the long run, it increases efficiency and reduces the risk of code failures.
6. Efficient Exception Management
Handling exceptions correctly is crucial to ensuring your software is robust and resilient. Some tips for good exception management include:
-
Specific Exceptions: whenever possible, throw specific exceptions instead of using generic Exception. This makes the code easier to understand and debug.
-
Avoid Silent Exceptions: never catch an exception without handling it properly or at least logging the error message. Ignoring exceptions can hide critical problems in the system.
-
try-with-resources: Use this approach when working with resources such as files and database connections. It ensures that these resources are automatically closed at the end of use, preventing memory leaks.
By following good exception handling practices, your code becomes more reliable and easier to maintain.
7. Adopt Design Patterns
Design Patterns are proven solutions to recurring problems in software development. In Java, some classic patterns that can help you structure your code more efficiently include:
-
Singleton: ensures that a class has only one instance and provides a global point of access to it.
-
Factory: allows the creation of objects without specifying the exact class to be instantiated. This makes it easier to extend the code without changing the parts that depend on this creation.
-
Strategy: allows you to change the behavior of an object at run time, without modifying the class that uses it.
These patterns are valuable for ensuring your code is scalable, flexible, and easy to understand.
Bonus: Use Code Quality Tools in Java
Code quality tools are essential for identifying problems in the code before they affect the operation of the application. For Java projects, consider using the following tools:
-
Linters: analyze the style and consistency of the code, ensuring that it follows Java best practices and conventions (example: Checkstyle).
-
Static Analyzers: detect potential errors, vulnerabilities and performance problems without the need to execute the code (example: SonarQube).
-
Automatic Formatters: ensure that the code is always formatted according to style conventions, such as the Google Java standard (example: Google Java Format).
References used:
- https://napoleon.com.br/glossario/o-que-e-java-naming-conventions/
- https://www.devmedia.com.br/programacao-orientada-a-objetos-com-java/18449
- https://www.macoratti.net/16/04/net_dry1.htm
- https://caffeinealgorithm.com/blog/comentarios-em-java
- https://www.devmedia.com.br/testes-automatizados-com-junit/30324
- https://blog.cubos.academy/java-boas-praticas-e-padroes-de-codigo/
- https://www.baeldung.com/java-try-with-resources
- https://devnit.medium.com/gerenciamento-de-exceções-em-java-e-spring-boot-melhores-práticas-a0395db28df7
- https://refactoring.guru/pt-br/design-patterns/java
The above is the detailed content of How to Improve Your Java Code: Development Practices and Patterns. For more information, please follow other related articles on the PHP Chinese website!