The Date object in JavaScript is used to work with dates and times. It provides methods to create, manipulate, and format date and time values.
Creating Dates
You can create a Date object in multiple ways:
- Current Date and Time:
const now = new Date(); console.log(now); // Current date and time
- Specific Date:
const specificDate = new Date(2024, 10, 21); // Year, Month (0-based), Day console.log(specificDate); // Thu Nov 21 2024
- From a String:
const fromString = new Date("2024-11-21T10:00:00"); console.log(fromString); // Thu Nov 21 2024 10:00:00 GMT
- From Timestamps (milliseconds since Unix epoch):
const fromTimestamp = new Date(1732231200000); console.log(fromTimestamp); // Thu Nov 21 2024 10:00:00 GMT
Common Methods
Getting Date and Time
|
Description |
Example | |||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
getFullYear() | Returns the year | date.getFullYear() -> 2024 | |||||||||||||||||||||||||||
getMonth() | Returns the month (0-11) | date.getMonth() -> 10 (November) | |||||||||||||||||||||||||||
getDate() | Returns the day of the month (1-31) | date.getDate() -> 21 | |||||||||||||||||||||||||||
getDay() | Returns the weekday (0-6, Sun=0) | date.getDay() -> 4 (Thursday) | |||||||||||||||||||||||||||
getHours() | Returns the hour (0-23) | date.getHours() -> 10 | |||||||||||||||||||||||||||
getMinutes() | Returns the minutes (0-59) | date.getMinutes() -> 0 | |||||||||||||||||||||||||||
getSeconds() | Returns the seconds (0-59) | date.getSeconds() -> 0 | |||||||||||||||||||||||||||
getTime() | Returns timestamp in milliseconds | date.getTime() -> 1732231200000 |
Method | Description | Example |
---|---|---|
setFullYear(year) | Sets the year | date.setFullYear(2025) |
setMonth(month) | Sets the month (0-11) | date.setMonth(0) -> January |
setDate(day) | Sets the day of the month | date.setDate(1) -> First day of the month |
setHours(hour) | Sets the hour (0-23) | date.setHours(12) |
setMinutes(minutes) | Sets the minutes (0-59) | date.setMinutes(30) |
setSeconds(seconds) | Sets the seconds (0-59) | date.setSeconds(45) |
Method | Description | Example |
---|---|---|
setFullYear(year) | Sets the year | date.setFullYear(2025) |
setMonth(month) | Sets the month (0-11) | date.setMonth(0) -> January |
setDate(day) | Sets the day of the month | date.setDate(1) -> First day of the month |
setHours(hour) | Sets the hour (0-23) | date.setHours(12) |
setMinutes(minutes) | Sets the minutes (0-59) | date.setMinutes(30) |
setSeconds(seconds) | Sets the seconds (0-59) | date.setSeconds(45) |
Formatting Dates
|
Description |
Example | |||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
toDateString() | Returns date as a human-readable string | date.toDateString() -> "Thu Nov 21 2024" | |||||||||||||||
toISOString() | Returns date in ISO format | date.toISOString() -> "2024-11-21T10:00:00.000Z" | |||||||||||||||
toLocaleDateString() | Returns date in localized format | date.toLocaleDateString() -> "11/21/2024" | |||||||||||||||
toLocaleTimeString() | Returns time in localized format | date.toLocaleTimeString() -> "10:00:00 AM" |
- Common Use Cases
const now = new Date(); console.log(now); // Current date and time
- Calculate Days Between Two Dates
- :
const specificDate = new Date(2024, 10, 21); // Year, Month (0-based), Day console.log(specificDate); // Thu Nov 21 2024
- Countdown Timer
- :
const fromString = new Date("2024-11-21T10:00:00"); console.log(fromString); // Thu Nov 21 2024 10:00:00 GMT
- Format Current Date
- :
const fromTimestamp = new Date(1732231200000); console.log(fromTimestamp); // Thu Nov 21 2024 10:00:00 GMT
- Find the Day of the Week
- :
const startDate = new Date("2024-11-01"); const endDate = new Date("2024-11-21"); const diffInTime = endDate - startDate; // Difference in milliseconds const diffInDays = diffInTime / (1000 * 60 * 60 * 24); // Convert to days console.log(diffInDays); // 20
- Check Leap Year
- :
const targetDate = new Date("2024-12-31T23:59:59"); setInterval(() => { const now = new Date(); const timeLeft = targetDate - now; // Milliseconds left const days = Math.floor(timeLeft / (1000 * 60 * 60 * 24)); const hours = Math.floor((timeLeft / (1000 * 60 * 60)) % 24); const minutes = Math.floor((timeLeft / (1000 * 60)) % 60); const seconds = Math.floor((timeLeft / 1000) % 60); console.log(`${days}d ${hours}h ${minutes}m ${seconds}s`); }, 1000);
Add/Subtract Days
:
- Pro Tips
const now = new Date(); const formatted = `${now.getFullYear()}-${now.getMonth() + 1}-${now.getDate()}`; console.log(formatted); // "2024-11-21"Use
- Date.now()
- to get the current timestamp directly without creating a Date object:
Be mindful of time zones
when working with dates across regions. Use libraries like Moment.js - or
Day.js for advanced handling.
0-indexed
The above is the detailed content of JavaScript Date Object Cheatsheet. For more information, please follow other related articles on the PHP Chinese website!
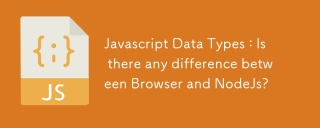
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
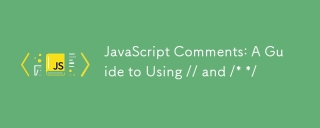
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
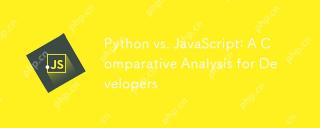
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
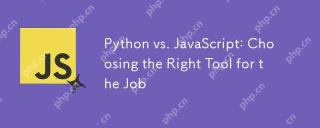
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
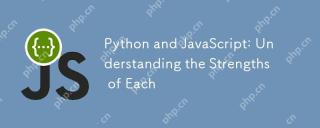
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
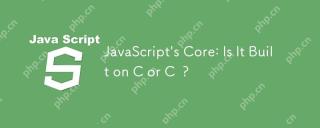
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
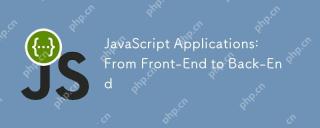
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
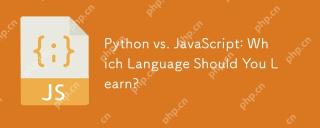
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
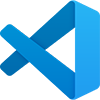
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
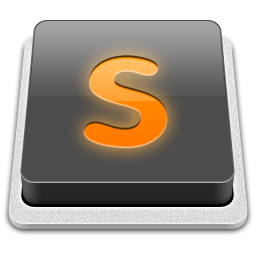
SublimeText3 Mac version
God-level code editing software (SublimeText3)
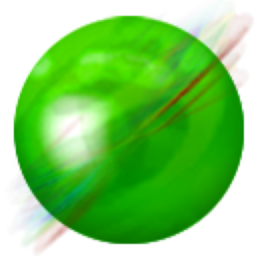
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
