Displaying Firebase Data in ListView with Score Property
When attempting to display both the User ID and Score from Firebase data in a ListView, the score value may initially be unavailable. Here's a comprehensive guide to addressing this issue and effectively presenting both properties.
Asynchronous Nature of Firebase API
The onDataChange() method used to retrieve data from Firebase is asynchronous, meaning it may be called before the Score objects are added to the list. To address this, declare the list within the onDataChange() method or employ techniques to handle asynchronous behavior effectively.
Data Structure and Type
Ensure that your Score class follows Java Naming Conventions for data members. Additionally, modify it as follows:
public class Score { private String userId; private String score; // ... [other class methods] }
Data Retrieval and Display Using String
To display data using the String class, utilize the code snippet below:
ListView listView = (ListView) findViewById(R.id.list_view); List<string> list = new ArrayList(); // Declare list within onDataChange() method ArrayAdapter<string> arrayAdapter = new ArrayAdapter(getActivity(), android.R.layout.simple_list_item_1, list); listView.setAdapter(arrayAdapter); DatabaseReference rootRef = FirebaseDatabase.getInstance().getReference(); DatabaseReference scoreRef = rootRef.child("score"); ValueEventListener eventListener = new ValueEventListener() { @Override public void onDataChange(DataSnapshot dataSnapshot) { for(DataSnapshot ds : dataSnapshot.getChildren()) { String userId = ds.child("userId").getValue(String.class); String score = ds.child("score").getValue(String.class); list.add(userId + " / " + score); } arrayAdapter.notifyDataSetChanged(); } // ... [onCancelled() method] }; scoreRef.addListenerForSingleValueEvent(eventListener);</string></string>
Data Retrieval and Display Using Score Class
To retrieve and display data using the Score class, employ the following code snippet:
ValueEventListener eventListener = new ValueEventListener() { @Override public void onDataChange(DataSnapshot dataSnapshot) { for(DataSnapshot ds : dataSnapshot.getChildren()) { Score score = ds.getValue(Score.class); String userId = score.getUserId(); String score = score.getScore(); list.add(userId + " / " + score); } arrayAdapter.notifyDataSetChanged(); } // ... [onCancelled() method] }; scoreRef.addListenerForSingleValueEvent(eventListener);
The above is the detailed content of How to Efficiently Display Firebase User IDs and Scores in a ListView?. For more information, please follow other related articles on the PHP Chinese website!
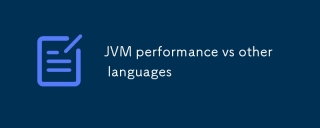
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
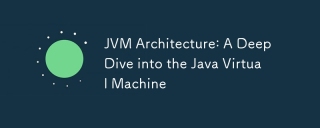
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
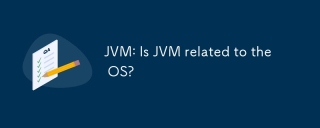
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
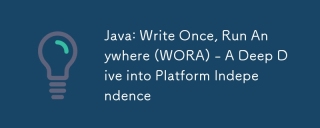
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
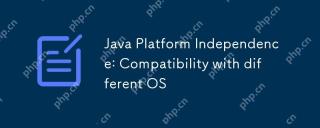
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
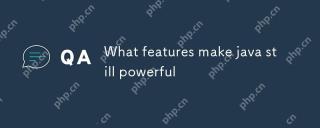
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
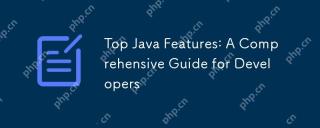
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
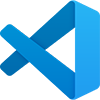
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
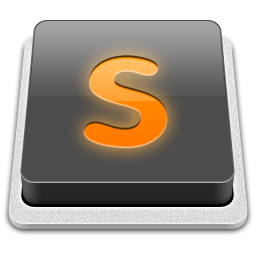
SublimeText3 Mac version
God-level code editing software (SublimeText3)
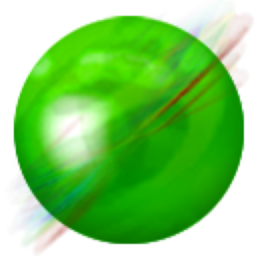
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
