


How to Sequence and Share Data in Promise Chains
Promises offer a powerful tool for managing asynchronous operations in JavaScript. As part of this, it becomes necessary to sequence operations and share data between them. Let's address the specific issue:
Chaining HTTP Requests and Data Sharing with Promises
In this scenario, you're using Promises to perform a series of HTTP requests, where the response from one request should be used as input for the next. The callhttp function handles these requests but needs to access data from previous requests to construct the next one. Specifically, you want to pass the API key obtained from the first request to subsequent requests.
Approaches for Data Sharing and Sequencing
There are several approaches to achieve this:
1. Chained Promises:
Chain the promises using then, passing intermediate data as arguments:
callhttp(url1, payload) .then(firstResponse => { // Use the data from firstResponse in the next request return callhttp(url2, payload, firstResponse.apiKey); }) .then(secondResponse => { // Use the combined data from firstResponse and secondResponse in the next request return callhttp(url3, payload, firstResponse.apiKey, secondResponse.userId); });
2. Higher Scope Assignment:
Assign intermediate results to variables in a higher scope:
var firstResponse; callhttp(url1, payload) .then(result => { firstResponse = result; return callhttp(url2, payload); }) .then(secondResponse => { // Use both firstResponse and secondResponse here });
3. Accumulate Results:
Store results in an accumulating object:
var results = {}; callhttp(url1, payload) .then(result => { results.first = result; return callhttp(url2, payload); }) .then(result => { results.second = result; return callhttp(url3, payload); }) .then(() => { // Use the accumulated results in results object });
4. Nested Promises:
Nest promises to maintain access to all previous results:
callhttp(url1, payload) .then(firstResponse => { // Use firstResponse here return callhttp(url2, payload) .then(secondResponse => { // Use both firstResponse and secondResponse here return callhttp(url3, payload); .then(thirdResponse => { // Use all three responses here }); }); });
5. Split with Promise.all():
If some requests can be made independently, break the chain into separate pieces and use Promise.all() to collect the results:
const first = callhttp(url1, payload); const second = callhttp(url2, payload); const third = callhttp(url3, payload); Promise.all([first, second, third]) .then(results => { // Use all three results here });
ES7 Async/Await:
In ES7, the async/await syntax streamlines the process of sequencing and handling promise results, providing a simpler and more readable code:
async function httpRequests() { const firstResponse = await callhttp(url1, payload); const secondResponse = await callhttp(url2, payload, firstResponse.apiKey); const thirdResponse = await callhttp(url3, payload, firstResponse.apiKey, secondResponse.userId); // Use all three responses here } httpRequests();
The above is the detailed content of How to Efficiently Sequence and Share Data Between Promises in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
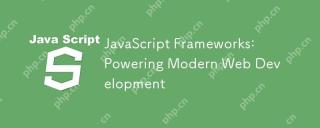
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
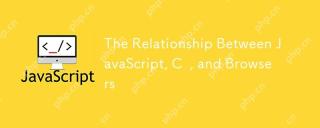
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
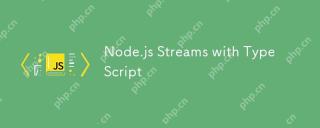
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
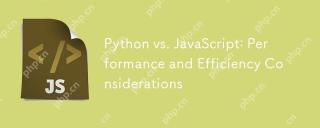
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
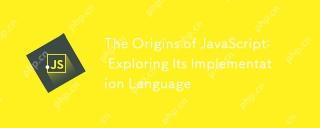
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
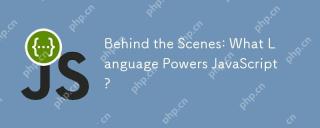
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
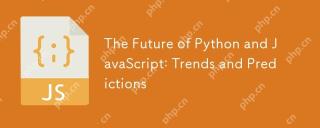
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
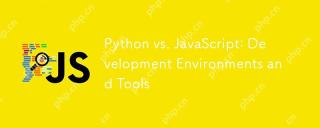
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
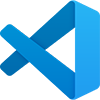
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use
