Lookup Functions in Go
Many network-related operations rely on DNS lookups to resolve hostnames to IP addresses and other information. Golang provides a set of convenient functions in the net package for performing DNS lookups, including LookupAddr and LookupTXT, which return the corresponding IP addresses or text records for a given hostname.
One limitation of these functions is that they typically rely on the system's resolvers configured in /etc/resolv.conf. While this is sufficient in most cases, there may be instances where you need to specify a specific DNS server to perform the lookup.
Lookup Functions with Custom DNS Server
Unfortunately, the standard Go library does not currently offer functions like LookupAddrWithServer or LookupTXTWithServer that allow you to specify a custom DNS server. However, there are several third-party libraries that provide this functionality, including the popular github.com/miekg/dns.
The following modified example from the answer given by @holys demonstrates how you can use the miekg/dns library to perform DNS lookups with a specified server:
package main import ( "fmt" "log" "github.com/miekg/dns" ) func main() { target := "example.com" server := "8.8.8.8" c := dns.Client{} m := dns.Msg{} m.SetQuestion(target, dns.TypeA) r, t, err := c.Exchange(&m, server+":53") if err != nil { log.Fatal(err) } fmt.Printf("Took %v", t) if len(r.Answer) == 0 { log.Fatal("No results") } for _, ans := range r.Answer { arecord := ans.(*dns.A) fmt.Printf("%s", arecord.A) } }
This example uses the github.com/miekg/dns library to perform an A record lookup for example.com using the specified DNS server at 8.8.8.8. By leveraging third-party libraries like miekg/dns, you can extend the functionality provided by the Go standard library to meet your specific requirements.
The above is the detailed content of How Can I Perform DNS Lookups in Go with a Custom DNS Server?. For more information, please follow other related articles on the PHP Chinese website!
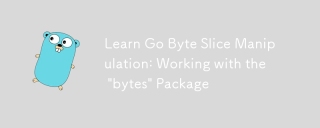
ThebytespackageinGoisessentialformanipulatingbytesliceseffectively.1)Usebytes.Jointoconcatenateslices.2)Employbytes.Bufferfordynamicdataconstruction.3)UtilizeIndexandContainsforsearching.4)ApplyReplaceandTrimformodifications.5)Usebytes.Splitforeffici
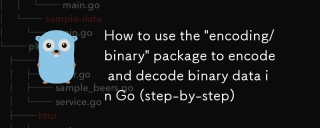
Tousethe"encoding/binary"packageinGoforencodinganddecodingbinarydata,followthesesteps:1)Importthepackageandcreateabuffer.2)Usebinary.Writetoencodedataintothebuffer,specifyingtheendianness.3)Usebinary.Readtodecodedatafromthebuffer,againspeci
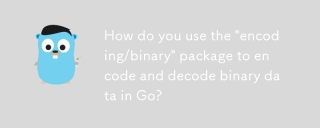
The encoding/binary package provides a unified way to process binary data. 1) Use binary.Write and binary.Read functions to encode and decode various data types such as integers and floating point numbers. 2) Custom types can be handled by implementing the binary.ByteOrder interface. 3) Pay attention to endianness selection, data alignment and error handling to ensure the correctness and efficiency of the data.

Go's strings package is not suitable for all use cases. It works for most common string operations, but third-party libraries may be required for complex NLP tasks, regular expression matching, and specific format parsing.
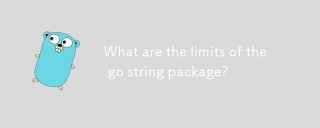
The strings package in Go has performance and memory usage limitations when handling large numbers of string operations. 1) Performance issues: For example, strings.Replace and strings.ReplaceAll are less efficient when dealing with large-scale string replacements. 2) Memory usage: Since the string is immutable, new objects will be generated every operation, resulting in an increase in memory consumption. 3) Unicode processing: It is not flexible enough when handling complex Unicode rules, and may require the help of other packages or libraries.
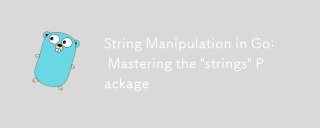
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
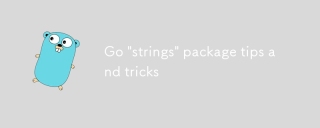
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
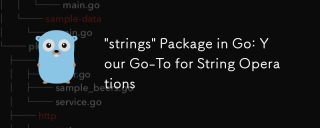
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
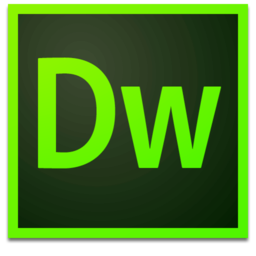
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
