How to wire one pane to another: Wiring output to paneWithList
To connect the output of the selected row in PaneWithList to the JTextPane in output, consider using the observer pattern. This design pattern involves creating an observable class (such as PaneWithList) that notifies its observers (such as output) when a property changes.
Implementing the Observer Pattern
1. Add a PropertyChangeListener to PaneWithList:
class PaneWithList extends JPanel { ... private PropertyChangeSupport propertyChangeSupport = new PropertyChangeSupport(this); ... // Notify observers when the selected row changes protected void fireSelectedRowChanged(int oldValue, int newValue) { propertyChangeSupport.firePropertyChange("SELECTED_ROW", oldValue, newValue); } // Add a property change listener public void addPropertyChangeListener(PropertyChangeListener listener) { propertyChangeSupport.addPropertyChangeListener(listener); } }
2. Create an observer class Output:
class Output extends JTextPane implements PropertyChangeListener { ... // Handle property change events from `PaneWithList` @Override public void propertyChange(PropertyChangeEvent e) { if (e.getPropertyName().equals("SELECTED_ROW")) { int row = (int) e.getNewValue(); String selectedItem = paneWithList.getSelectedValue(); // Get the selected item from `PaneWithList` append(selectedItem + "\n"); // Display the selected item in the text pane } } }
3. Establish the Observer Relationship:
In your Main class, add an observer to PaneWithList and connect it to the Output object.
import java.beans.PropertyChangeListener; public class Main { ... public static void main(String[] args) { ... paneWithList.addPropertyChangeListener(new Output()); // Connect to `Output` ... } }
Now, when the selected row in PaneWithList changes, Output will be notified and will update its text accordingly.
The above is the detailed content of How to Wire a JTextPane to Update with PaneWithList's Selected Row?. For more information, please follow other related articles on the PHP Chinese website!
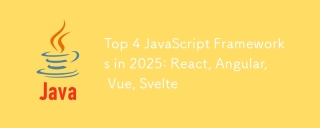
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
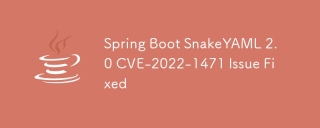
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
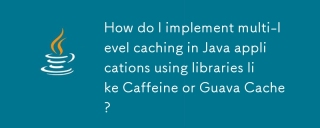
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
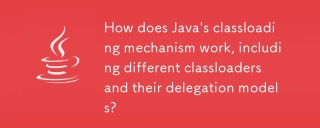
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
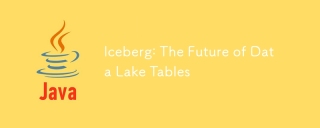
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
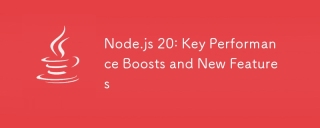
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
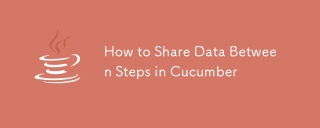
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
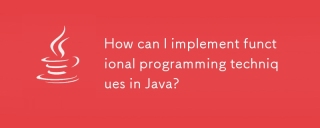
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
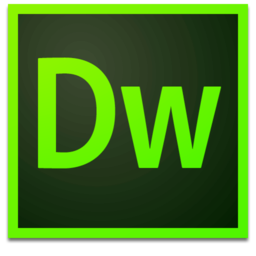
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
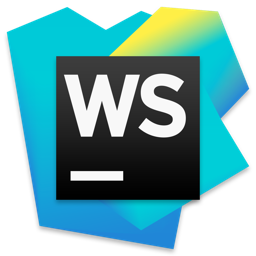
WebStorm Mac version
Useful JavaScript development tools
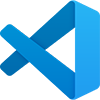
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
