Mass Replacement of Strings in JavaScript
The task of replacing multiple words in a string with different ones arises frequently in programming. Consider the following scenario:
const str = "I have a cat, a dog, and a goat.";
We want to transform this string into "I have a dog, a goat, and a cat" by replacing "cat" with "dog", "dog" with "goat", and "goat" with "cat".
Problem: Consecutive Replacements Lead to Incorrect Output
A naive approach would involve using the replace() method multiple times:
str = str.replace(/cat/gi, "dog"); str = str.replace(/dog/gi, "goat"); str = str.replace(/goat/gi, "cat");
Unfortunately, this code produces the incorrect result: "I have a cat, a cat, and a cat". This is because the second and third replacements overwrite the changes made by the previous ones.
Solution: Using a Map and a Custom Replacement Function
To address this issue, we need a more comprehensive approach that replaces all the target words simultaneously. This can be achieved by defining a map object that contains the replacements and using a custom replacement function:
const mapObj = { cat: "dog", dog: "goat", goat: "cat", }; const str = str.replace(/cat|dog|goat/gi, (matched) => { return mapObj[matched]; });
Dynamically Generating the Regex Pattern
To make the solution more generalizable, we can dynamically generate the regular expression pattern based on the keys of the map object:
const re = new RegExp(Object.keys(mapObj).join("|"), "gi"); const str = str.replace(re, (matched) => { return mapObj[matched]; });
Reusable Function for Mass String Replacement
Finally, we can encapsulate this approach into a reusable function:
const replaceAll = (str, mapObj) => { const re = new RegExp(Object.keys(mapObj).join("|"), "gi"); return str.replace(re, (matched) => { return mapObj[matched.toLowerCase()]; }); };
This function takes a string and a map of replacements and returns the transformed string.
In summary, there are several ways to replace multiple strings with multiple other strings in JavaScript. The solution presented here uses a map object and a custom replacement function to ensure that all replacements are performed simultaneously, resulting in the desired output accurately.
The above is the detailed content of How to Efficiently Replace Multiple Strings in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
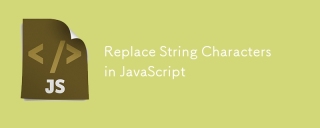
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
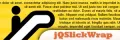
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
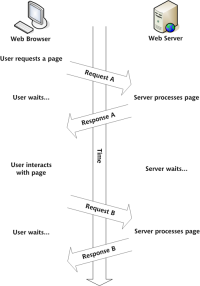
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
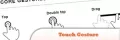
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
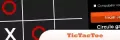
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
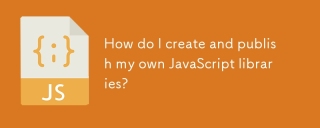
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
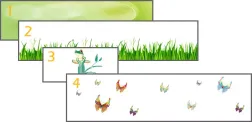
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
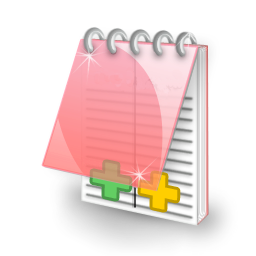
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
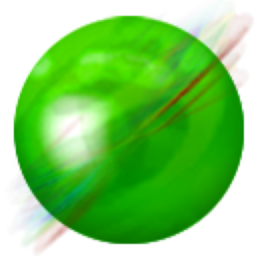
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
