Why Doesn\'t My JPanel Update After Adding or Moving Images in a Puzzle Game?
JPanel in Puzzle Game Not Updating
In a simple puzzle game, images representing tiles are randomly placed on a grid. Each image has 'place' and 'number' attributes indicating its current and desired position. The game logic correctly swaps images that match in 'number' and updates their 'place' attributes. However, adding the updated images to the JPanel does not update the displayed grid.
To address this issue, we'll modify the addComponents() method to properly refresh the JPanel:
public void addComponents(Img[] im){ this.removeAll(); for(int i=0; i<p>By invoking revalidate() and repaint(), we force the JPanel to recalculate its layout and update the display with the new images.</p><p>Alternatively, consider the following code example, which utilizes a more efficient approach:</p><pre class="brush:php;toolbar:false">import java.awt.Container; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.GridLayout; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; import javax.swing.ImageIcon; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.SwingUtilities; import javax.swing.Timer; import javax.swing.WindowConstants; public class PuzzleGame { private static final int N = 4; private final JPanel panel = new JPanel(new GridLayout(N, N)); private final JButton[][] buttons = new JButton[N][N]; private final BufferedImage image; private PuzzleGame() throws IOException { image = ImageIO.read(new File("image.jpg")); createButtons(); JFrame frame = new JFrame(); frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); frame.add(panel); frame.pack(); frame.setVisible(true); // Timer to simulate image swapping Timer timer = new Timer(1000, new ActionListener() { @Override public void actionPerformed(ActionEvent e) { shuffleButtons(); } }); timer.start(); } private void createButtons() { int count = 0; for (int i = 0; i <p>This code creates sliced image buttons and stores them in a 4x4 grid. A timer stimulates image shuffling by moving the top-right button to the first position. The revalidate() and repaint() methods ensure the UI updates correctly after each button movement.</p>
The above is the detailed content of Why Doesn\'t My JPanel Update After Adding or Moving Images in a Puzzle Game?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
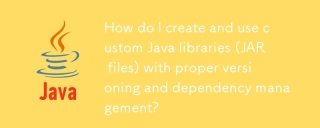
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
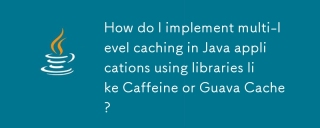
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
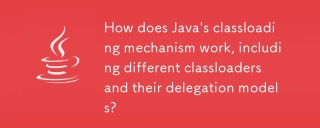
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
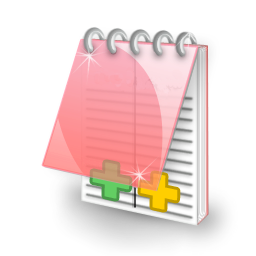
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment