


Which is Better: Using the `Calendar` Class or Custom Logic for Leap Year Calculation in Java?
Optimizing Leap Year Calculations in Java
When writing code to calculate leap years, there are multiple approaches to consider. The provided code implementation in the book uses the ACM Java Task Force's library, while your own code relies on explicit conditions. Let's explore which approach is preferable.
Approach 1: Using the Calendar Class
The ideal approach to calculate leap years is by leveraging the built-in Calendar class. The code below showcases this method:
public static boolean isLeapYear(int year) { Calendar cal = Calendar.getInstance(); cal.set(Calendar.YEAR, year); return cal.getActualMaximum(Calendar.DAY_OF_YEAR) > 365; }
This implementation is accurate and efficient as it utilizes the Calendar class that encapsulates the functionality for date and time management.
Approach 2: Implementing Custom Leap Year Logic
If you prefer to define your own logic, you can use the following code:
public static boolean isLeapYear(int year) { if (year % 4 != 0) { return false; } else if (year % 400 == 0) { return true; } else if (year % 100 == 0) { return false; } else { return true; } }
This code follows the rules that define leap years: years divisible by 4 are leap years, except for those divisible by 100. However, if a year is divisible by 400, it is considered a leap year regardless of divisibility by 100.
Ultimately, your choice of method depends on your application's specific requirements and performance needs. If accuracy and efficiency are paramount, using the Calendar class is recommended. If you prioritize a custom implementation, the provided code snippet should suffice.
The above is the detailed content of Which is Better: Using the `Calendar` Class or Custom Logic for Leap Year Calculation in Java?. For more information, please follow other related articles on the PHP Chinese website!
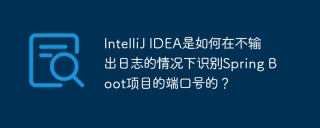
Start Spring using IntelliJIDEAUltimate version...
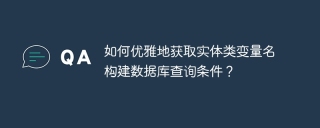
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
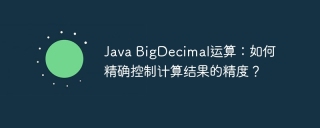
Java...
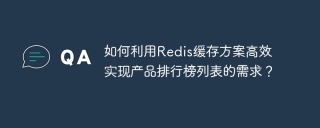
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
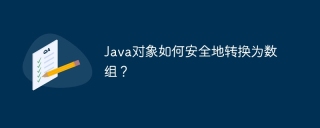
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
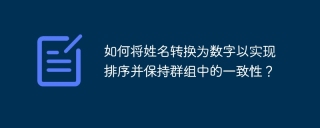
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
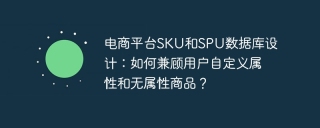
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
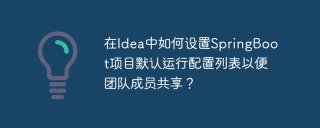
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
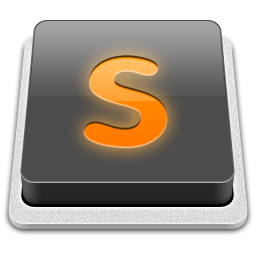
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool