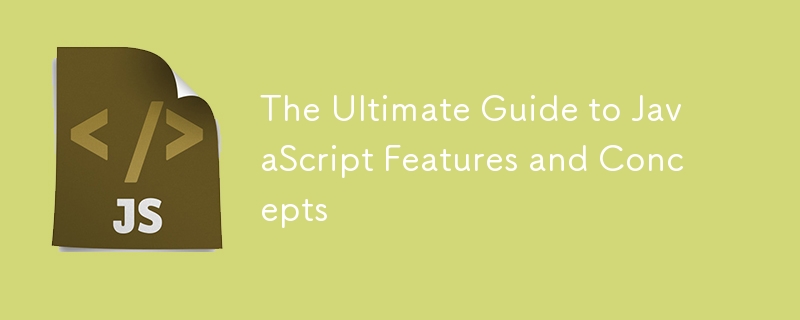
JavaScript is a versatile language with a rich set of features. Whether you're a beginner or an advanced developer, mastering these concepts can help you write efficient and robust code. Below is a comprehensive overview of key JavaScript topics and features:
Variables and Data Types
Declaring Variables
-
var: Function-scoped, can be redeclared, and hoisted.
-
let: Block-scoped, cannot be redeclared within the same block.
-
const: Block-scoped, immutable references (values can still be mutable if they are objects).
Primitive Types
-
string, number, boolean, null, undefined, symbol, bigint.
Reference Types
- Objects, Arrays, and Functions.
Control Structures
-
if, else, else if: Conditional execution.
-
switch: Cleaner syntax for multiple conditions.
Loops
-
for, while, do-while: For iterative tasks.
-
for…of: Iterates over iterable objects like arrays or strings.
-
for…in: Iterates over object properties.
Functions
Types of Functions
-
Function Declarations:
function greet() { console.log("Hello!"); }
-
Function Expressions:
const greet = function() { console.log("Hello!"); };
-
Arrow Functions:
const greet = () => console.log("Hello!");
Advanced Concepts
-
Immediately Invoked Function Expression (IIFE):
(function() { console.log("IIFE!"); })();
-
Higher-Order Functions: Functions that take other functions as arguments or return them.
-
Callback Functions: Functions passed as arguments for asynchronous or event-driven behavior.
Promises
Promises are used for handling asynchronous operations.
Key Methods:
-
Promise.all(): Resolves when all promises resolve.
-
Promise.resolve(): Returns a resolved promise.
-
Promise.then(): Handles resolved values.
-
Promise.any(): Resolves with the first fulfilled promise.
-
Promise.race(): Resolves/rejects with the first promise to settle.
-
Promise.reject(): Returns a rejected promise.
Async/Await
Syntactic sugar over Promises for writing asynchronous code that looks synchronous.
Closures
A closure is a function that retains access to its parent scope even after the parent function has returned.
function greet() { console.log("Hello!"); }
Scope and Hoisting
Types of Scope
-
Global Scope: Variables accessible everywhere.
-
Function Scope: Variables declared inside a function.
-
Block Scope: Variables declared with let and const inside a block.
Hoisting
- Variables declared with var are hoisted but initialized as undefined.
- Function declarations are hoisted with their definitions.
Event Loop and Task Queue
- The event loop manages asynchronous code execution by moving tasks from the task queue (macrotasks) or microtasks to the call stack.
Advanced Concepts
Debouncing and Throttling
-
Debouncing: Delays execution until after a pause in events.
-
Throttling: Ensures execution happens only once in a specified interval.
Currying
Converts a function with multiple arguments into a series of functions taking one argument each.
Built-In Methods
Array Methods
- Modifying Arrays: push(), pop(), shift(), unshift(), splice().
- Non-Mutating Methods: map(), filter(), reduce(), forEach().
Object Methods
-
Object.keys(), Object.values(), Object.entries().
-
Object.assign(), Object.freeze(), Object.seal().
String Methods
-
charAt(), includes(), slice(), split(), trim().
Date Methods
-
Date.now(), getDate(), getMonth(), setFullYear().
Prototypes and Classes
Prototypes
- Every JavaScript object has a prototype, which allows for inheritance.
- Understanding the prototype chain is crucial for object-oriented JavaScript.
Classes
- ES6 introduced class syntax as a syntactic sugar over prototypes.
- Features include constructors, inheritance, getters/setters, and static methods.
Error Handling
-
try...catch...finally: For catching runtime errors.
-
Custom Errors: Create error classes for better error handling.
Event Handling
- Adding event listeners: addEventListener().
- Preventing default behavior: event.preventDefault().
- Event delegation for efficient DOM manipulation.
Modern JavaScript Features
function greet() { console.log("Hello!"); }
const greet = function() { console.log("Hello!"); };
-
Spread and Rest Operators:
const greet = () => console.log("Hello!");
Miscellaneous Topics
-
LocalStorage and SessionStorage: For client-side data persistence.
-
Regular Expressions (RegExp): Pattern matching in strings.
-
Generators: Functions that yield values lazily.
-
JavaScript Proxy: Intercept and redefine fundamental operations.
-
WeakMap and WeakSet: Optimized for memory management.
-
Service Workers: Enable offline functionality for PWAs.
-
JSON: Parsing and stringifying data for APIs.
This guide attempts to cover a vast range of topics in JavaScript, but there’s always more to explore. If I missed any feature or you’d like more details, feel free to share your feedback!
The above is the detailed content of The Ultimate Guide to JavaScript Features and Concepts. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn