


How Can I Efficiently Spawn Multiple Instances of the Same Object Concurrently in Python?
Spawning Multiple Instances of the Same Object Concurrently in Python
When developing games or other interactive applications, the need to spawn multiple instances of the same object concurrently often arises. In Python, however, this task can present a challenge if not approached correctly.
Time-Based Generation
Timing is crucial when it comes to spawning objects concurrently. Instead of using sleep() or similar approaches that pause the program, a more efficient method involves using time measurements within the application loop.
Consider the following example:
time_interval = 500 # interval in milliseconds next_object_time = 0 object_list = [] while run: # ... other game logic current_time = pygame.time.get_ticks() if current_time > next_object_time: next_object_time += time_interval object_list.append(Object())
Here, we measure the time elapsed using pygame.time.get_ticks(). We define a time interval and check if the current time has exceeded the next object spawn time. If so, we create a new object and update the next spawn time.
Event-Based Generation
Another approach is to use the pygame.time module's event system. By defining a custom event with a time interval, we can have it trigger repeatedly, spawning new objects as needed.
time_interval = 500 # interval in milliseconds timer_event = pygame.USEREVENT+1 pygame.time.set_timer(timer_event, time_interval) object_list = [] while run: # ... other game logic for event in pygame.event.get(): if event.type == timer_event: object_list.append(Object())
In this scenario, we set up a timer event and handle it within the event loop. When the timer event is triggered, it signifies that it's time to spawn a new object.
Conclusion
By understanding the nuances of time and event handling in Python, you can spawn multiple instances of the same object concurrently, creating dynamic and engaging game experiences or other applications.
The above is the detailed content of How Can I Efficiently Spawn Multiple Instances of the Same Object Concurrently in Python?. For more information, please follow other related articles on the PHP Chinese website!
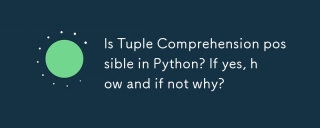
Article discusses impossibility of tuple comprehension in Python due to syntax ambiguity. Alternatives like using tuple() with generator expressions are suggested for creating tuples efficiently.(159 characters)
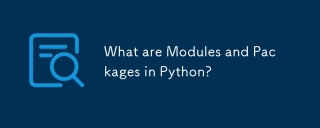
The article explains modules and packages in Python, their differences, and usage. Modules are single files, while packages are directories with an __init__.py file, organizing related modules hierarchically.
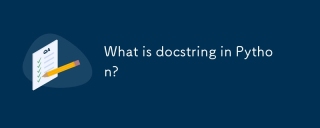
Article discusses docstrings in Python, their usage, and benefits. Main issue: importance of docstrings for code documentation and accessibility.
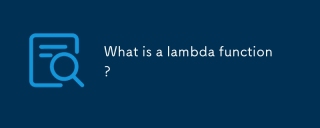
Article discusses lambda functions, their differences from regular functions, and their utility in programming scenarios. Not all languages support them.
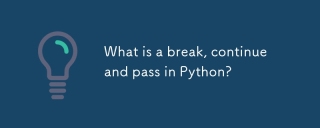
Article discusses break, continue, and pass in Python, explaining their roles in controlling loop execution and program flow.
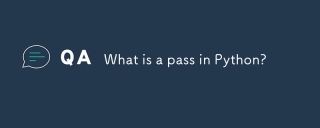
The article discusses the 'pass' statement in Python, a null operation used as a placeholder in code structures like functions and classes, allowing for future implementation without syntax errors.
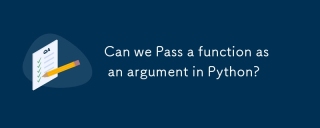
Article discusses passing functions as arguments in Python, highlighting benefits like modularity and use cases such as sorting and decorators.
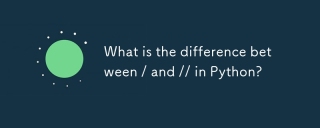
Article discusses / and // operators in Python: / for true division, // for floor division. Main issue is understanding their differences and use cases.Character count: 158


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
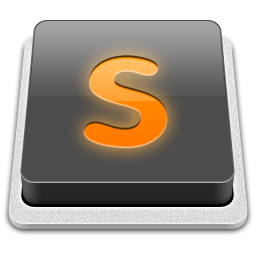
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!
