


How to Efficiently Find Common and Unique Elements in Two String Lists Using Java?
Comparing Two Lists
Given two lists of strings, the objective is to determine the number of common elements and identify both the shared and unique elements. One possible approach is to leverage Java's ArrayList and HashSet classes.
Using ArrayList:
The ArrayList class provides a convenient method called retainAll. When invoked with another collection, it removes the elements from the calling list that are not present in the argument collection. This can be utilized to identify common elements.
import java.util.ArrayList; ArrayList<string> list1 = new ArrayList(); list1.add("milan"); list1.add("dingo"); list1.add("elpha"); list1.add("hafil"); list1.add("meat"); list1.add("iga"); list1.add("neeta.peeta"); ArrayList<string> list2 = new ArrayList(); list2.add("hafil"); list2.add("iga"); list2.add("binga"); list2.add("mike"); list2.add("dingo"); list1.retainAll(list2); System.out.println("Common elements: " + list1);</string></string>
Using HashSet:
Similar to ArrayList, the HashSet class can be used to eliminate duplicates. By utilizing the addAll and removeAll methods, you can compute both common and unique elements.
import java.util.HashSet; HashSet<string> set1 = new HashSet(list1); HashSet<string> set2 = new HashSet(list2); // Common elements HashSet<string> common = new HashSet(set1); common.retainAll(set2); System.out.println("Common elements: " + common); // Unique elements HashSet<string> unique = new HashSet(); unique.addAll(set1); unique.addAll(set2); unique.removeAll(common); System.out.println("Unique elements: " + unique);</string></string></string></string>
These approaches offer efficient ways to compare lists and extract the desired information. Feel free to customize the code to meet your specific requirements.
The above is the detailed content of How to Efficiently Find Common and Unique Elements in Two String Lists Using Java?. For more information, please follow other related articles on the PHP Chinese website!
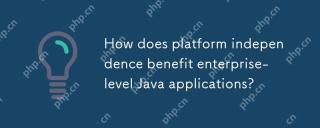
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
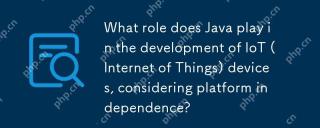
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
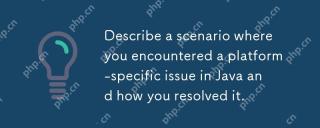
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
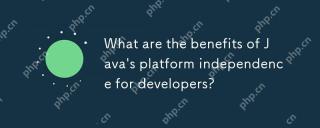
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
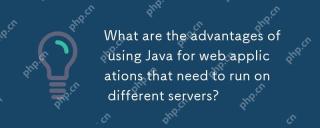
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
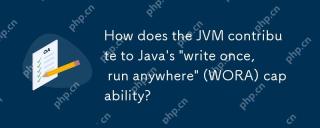
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
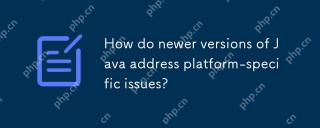
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
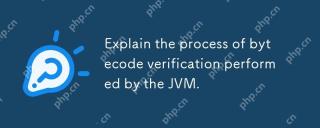
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
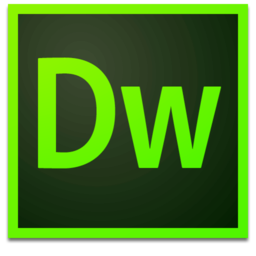
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
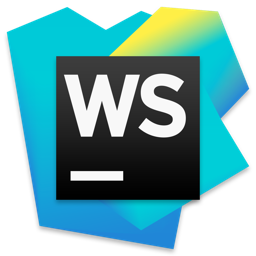
WebStorm Mac version
Useful JavaScript development tools
