Efficient Comparison of Unordered Lists
Comparing unordered lists, also known as bags, can be a challenging task, especially when working with objects instead of simple data types like integers. Here are three efficient approaches to tackle this problem:
1. Counter Method (O(n))
For objects that are hashable (i.e., can be converted to a unique hash value), the Counter() method from Python's collections module can be used. It creates a dictionary-like object where keys are the elements of the list and values are their respective counts. Comparing two lists using this approach has a linear time complexity of O(n), where n is the length of the lists.
import collections def compare(s, t): return collections.Counter(s) == collections.Counter(t)
2. Sorted Method (O(n log n))
If the objects in the list are comparable (i.e., they have a defined order), sorting the lists can provide an efficient comparison mechanism. The sorted() method in Python sorts the elements in the list, making it easy to determine if the two lists contain the same elements in any order. This approach has a time complexity of O(n log n), where n is the length of the lists.
def compare(s, t): return sorted(s) == sorted(t)
3. Equality Comparison (O(n * n))
If the objects are neither hashable nor orderable, a straightforward approach is to perform equality comparisons between the elements of the two lists. For each element in the first list, we remove it from the second list. If any element in the first list is not found in the second list, the comparison fails. This approach has a worst-case time complexity of O(n * n), where n is the length of the lists.
def compare(s, t): t = list(t) # make a mutable copy try: for elem in s: t.remove(elem) except ValueError: return False return not t
By utilizing these efficient comparison techniques, you can effectively compare unordered lists of objects, whether they are hashable, orderable, or neither.
The above is the detailed content of How to Efficiently Compare Unordered Lists of Objects?. For more information, please follow other related articles on the PHP Chinese website!
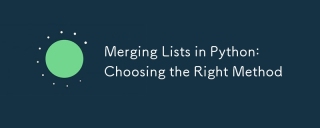
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
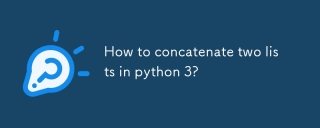
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
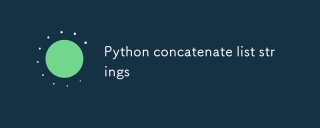
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
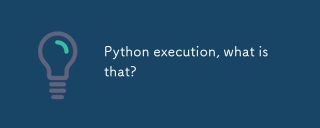
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
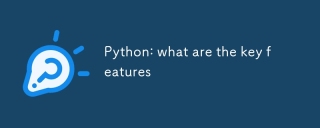
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
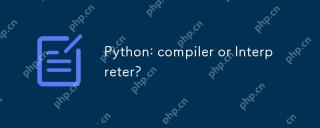
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
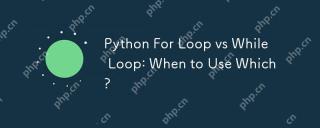
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
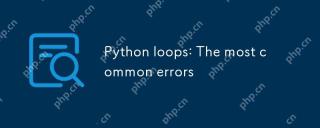
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
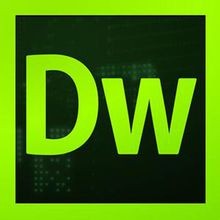
Dreamweaver CS6
Visual web development tools
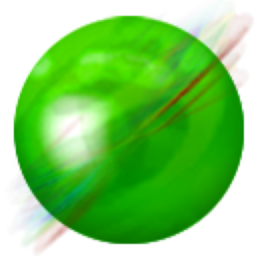
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
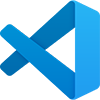
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
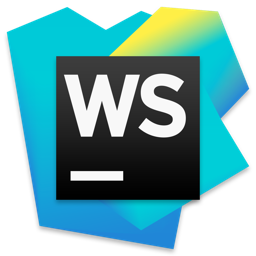
WebStorm Mac version
Useful JavaScript development tools
