Python List Sort in Descending Order
Sorting a list in descending order becomes necessary when you need to prioritize elements based on their magnitude. To achieve this in Python, we delve into the details of the built-in sorting function.
How to Sort a List in Descending Order
Python provides a straightforward way to sort a list in descending order using the sorted() function:
sorted_timestamps = sorted(timestamps, reverse=True)
The reverse argument, set to True, reverses the sorting order, effectively sorting the list in descending order.
Alternatively, you can modify the original list in-place with the sort() method:
timestamps.sort(reverse=True)
Example
Consider the following list of timestamps:
timestamps = [ "2010-04-20 10:07:30", "2010-04-20 10:07:38", "2010-04-20 10:07:52", "2010-04-20 10:08:22", "2010-04-20 10:08:22", "2010-04-20 10:09:46", "2010-04-20 10:10:37", "2010-04-20 10:10:58", "2010-04-20 10:11:50", "2010-04-20 10:12:13", "2010-04-20 10:12:13", "2010-04-20 10:25:38" ]
The sorted version of this list in descending order is:
["2010-04-20 10:25:38", "2010-04-20 10:12:13", "2010-04-20 10:12:13", "2010-04-20 10:11:50", "2010-04-20 10:10:58", "2010-04-20 10:10:37", "2010-04-20 10:09:46", "2010-04-20 10:08:22", "2010-04-20 10:08:22", "2010-04-20 10:07:52", "2010-04-20 10:07:38", "2010-04-20 10:07:30"]
Documentation
For further insights, refer to the official Python documentation on sorting:
- [Sorting HOW TO](https://docs.python.org/3/howto/sorting.html)
The above is the detailed content of How do I sort a Python list in descending order?. For more information, please follow other related articles on the PHP Chinese website!
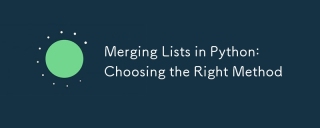
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
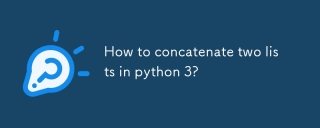
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
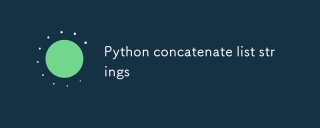
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
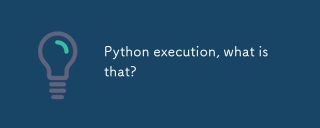
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
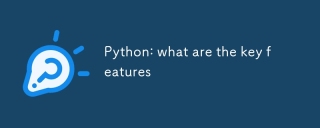
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
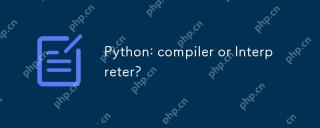
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
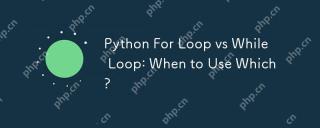
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
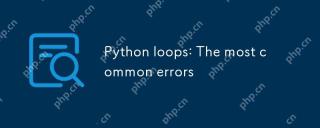
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
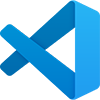
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
