


Troubleshooting "TypeError: 'str' does not support the buffer interface" in Python GZIP Compression
When compressing strings using Python's gzip module, developers may encounter the error "TypeError: 'str' does not support the buffer interface." This error stems from the transition from Python 2.x to Python 3.x, where the string data type has changed.
To resolve this issue, it is necessary to encode the string into bytes before writing it to the output file. The following code snippet corrects the code provided in the question:
plaintext = input("Please enter the text you want to compress").encode('UTF-8') filename = input("Please enter the desired filename") with gzip.open(filename + ".gz", "wb") as outfile: outfile.write(plaintext)
By encoding the plaintext into bytes, we ensure compatibility with the revised string data type in Python 3.x. Additionally, to avoid confusion, it is recommended to use different variable names for the input and output files, as "plaintext" and "filename" are reserved words in Python.
For example, the following code demonstrates how to effectively compress Polish text with UTF-8 encoding:
text = 'Polish text: ąćęłńóśźżĄĆĘŁŃÓŚŹŻ' filename = 'foo.gz' with gzip.open(filename, 'wb') as outfile: outfile.write(bytes(text, 'UTF-8')) with gzip.open(filename, 'r') as infile: decompressed_text = infile.read().decode('UTF-8') print(decompressed_text)
The above is the detailed content of How to Fix \'TypeError: \'str\' does not support the buffer interface\' in Python GZIP Compression?. For more information, please follow other related articles on the PHP Chinese website!
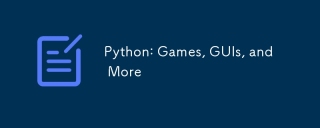
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
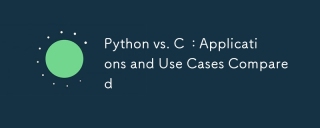
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
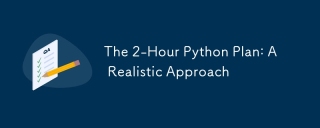
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
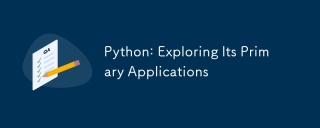
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
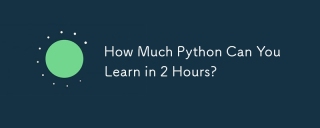
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
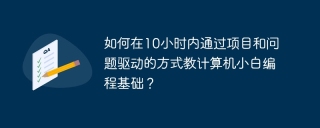
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
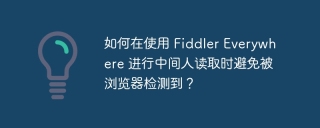
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
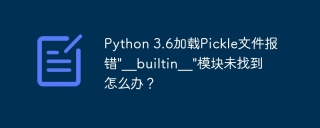
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
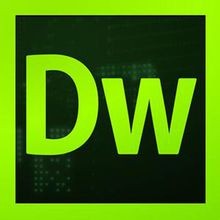
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.