


What\'s the Most Efficient Way to Perform Element-Wise Addition of Lists in Python?
Element-Wise Addition of Lists: A Pythonic Approach
Adding two lists element-wise can be performed effortlessly in Python using several built-in functions. Here's how to achieve this without cumbersome iterations:
Using map() with operator.add:
from operator import add result = list(map(add, list1, list2))
The map() function applies the add function to each corresponding element in list1 and list2, returning a list of the results.
Alternatively, using zip() with a list comprehension:
result = [sum(x) for x in zip(list1, list2)]
The zip() function pairs up the elements from list1 and list2 into a sequence of tuples. The list comprehension then calculates the sum of each tuple, producing the element-wise addition.
Performance Comparisons:
To compare the efficiency of these approaches, we conducted timing tests on large lists (100,000 elements):
>>> from itertools import izip >>> list2 = [4, 5, 6] * 10 ** 5 >>> list1 = [1, 2, 3] * 10 ** 5 >>> %timeit from operator import add; map(add, list1, list2) 10 loops, best of 3: 44.6 ms per loop >>> %timeit from itertools import izip; [a + b for a, b in izip(list1, list2)] 10 loops, best of 3: 71 ms per loop >>> %timeit [a + b for a, b in zip(list1, list2)] 10 loops, best of 3: 112 ms per loop >>> %timeit from itertools import izip; [sum(x) for x in izip(list1, list2)] 1 loops, best of 3: 139 ms per loop >>> %timeit [sum(x) for x in zip(list1, list2)] 1 loops, best of 3: 177 ms per loop
As these results demonstrate, the map() approach using operator.add is the fastest for large lists.
The above is the detailed content of What\'s the Most Efficient Way to Perform Element-Wise Addition of Lists in Python?. For more information, please follow other related articles on the PHP Chinese website!
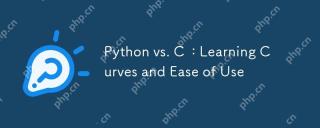
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
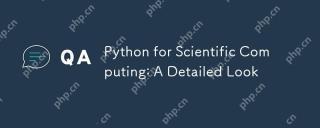
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
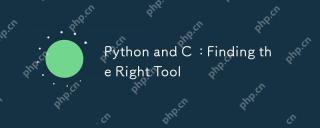
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
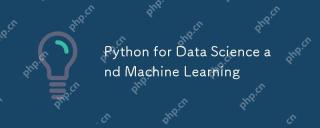
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
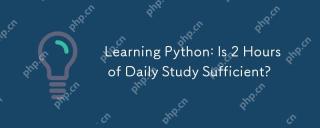
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
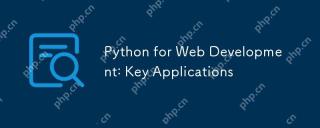
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
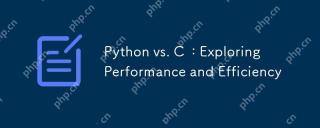
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
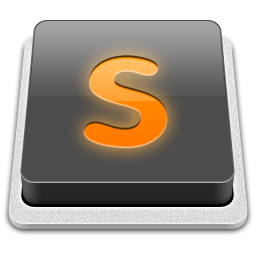
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.