


How Can I Read File Contents Client-Side in JavaScript Across Different Browsers?
Reading File Contents on the Client-Side in JavaScript: A Cross-Browser Solution
Introduction
Reading file contents on the client-side in a web browser can be a useful technique for various applications. While there are solutions for certain browsers like Firefox and Internet Explorer, achieving cross-browser compatibility can be challenging. This article explores different approaches for reading file contents across multiple browsers.
Mozilla File API
Firefox and Internet Explorer leverage the Mozilla File API to enable file reading. The API provides access to the file's name, size, and its binary contents. Using this API, developers can fetch the file contents as follows:
function getFileContents() { var fileForUpload = document.forms[0].fileForUpload; var fileName = fileForUpload.value; if (fileForUpload.files) { var fileContents = fileForUpload.files.item(0).getAsBinary(); document.forms[0].fileContents.innerHTML = fileContents; } else { // Handle other browsers with different file reading methods } }
IE File Reading
In Internet Explorer, the ActiveXObject library can be used for file reading. Here's how:
function ieReadFile(filename) { try { var fso = new ActiveXObject("Scripting.FileSystemObject"); var fh = fso.OpenTextFile(filename, 1); var contents = fh.ReadAll(); fh.Close(); return contents; } catch (Exception) { return "Cannot open file :("; } }
WebKit (Safari and Chrome)
Currently, WebKit browsers (such as Safari and Chrome) do not natively support file reading. To address this, you can either:
- Submit a patch to the WebKit project proposing the inclusion of the Mozilla File API.
- Propose the API's inclusion in HTML 5 through the WHATWG mailing list. Once incorporated into the standard, cross-browser compatibility would be enhanced.
File API
Since the initial introduction of the Mozilla File API, the File API has been formalized as a standard and implemented in most modern browsers. It offers a more standardized and robust approach to file reading, including support for asynchronous reading, binary file handling, and encoding decoding. Here's how you can use the File API:
var file = document.getElementById("fileForUpload").files[0]; if (file) { var reader = new FileReader(); reader.readAsText(file, "UTF-8"); reader.onload = function (evt) { document.getElementById("fileContents").innerHTML = evt.target.result; } reader.onerror = function (evt) { document.getElementById("fileContents").innerHTML = "error reading file"; } }
Conclusion
While native file reading support varies across browsers, the File API has emerged as a standardized solution for cross-browser file handling. By leveraging this API, developers can efficiently read file contents on the client-side, opening up possibilities for innovative web applications.
The above is the detailed content of How Can I Read File Contents Client-Side in JavaScript Across Different Browsers?. For more information, please follow other related articles on the PHP Chinese website!
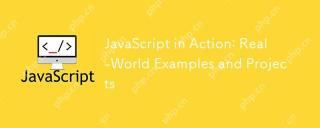
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
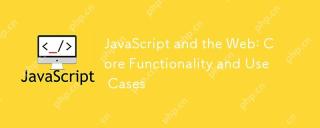
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
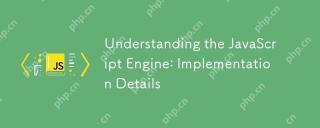
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
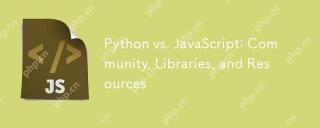
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
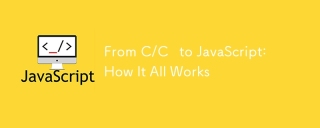
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
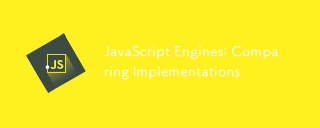
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
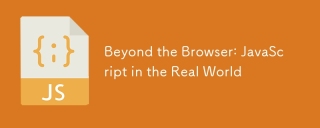
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor