Reallocating Memory in C
When working with dynamic memory allocation in C , developers often encounter the need to reallocate memory to accommodate changes in data size. In contrast to languages like C, C does not provide an explicit realloc function.
Considering Alternatives
Deleting the current pointer and allocating a new one with a larger size is not an optimal solution due to its inefficiency. Below is a better approach using the standard library.
Using Standard Templatized Library (STL) Vectors
STL vectors offer a convenient way to handle dynamic memory allocation and resizing. They provide efficient reallocation capabilities through their resize member function. Here's how to use vectors for reallocation:
Code Conversion:
// Old C code using realloc Type* t = (Type*)malloc(sizeof(Type)*n) memset(t, 0, sizeof(Type)*m) // New C++ code using std::vector std::vector<type> t(n, 0); // Resizing in C using realloc t = (Type*)realloc(t, sizeof(Type) * n2); // Resizing in C++ using vector::resize t.resize(n2);</type>
Calling Function with Vectors:
To pass a vector into a function, use the following syntax:
Foo(&t[0]); // Instead of Foo(t)
This ensures compatibility with function arguments expecting pointer arguments.
Advantages of STL Vectors
Using STL vectors for memory reallocation offers several advantages:
- Efficiency: Vectors perform reallocation efficiently through their optimized low-level implementation.
- Automatic Memory Management: Vectors handle memory allocation and deallocation automatically, simplifying memory management tasks.
- Flexibility: Vectors can hold objects of any type, making them highly versatile for various data structures.
The above is the detailed content of How Can C Developers Efficiently Reallocate Memory?. For more information, please follow other related articles on the PHP Chinese website!
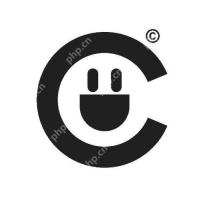
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
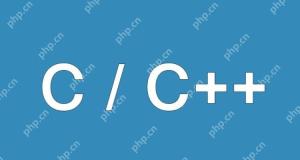
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
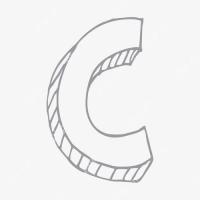
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
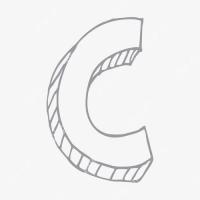
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
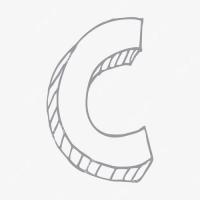
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
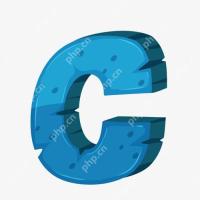
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.
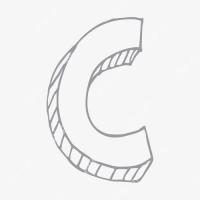
DMA in C refers to DirectMemoryAccess, a direct memory access technology, allowing hardware devices to directly transmit data to memory without CPU intervention. 1) DMA operation is highly dependent on hardware devices and drivers, and the implementation method varies from system to system. 2) Direct access to memory may bring security risks, and the correctness and security of the code must be ensured. 3) DMA can improve performance, but improper use may lead to degradation of system performance. Through practice and learning, we can master the skills of using DMA and maximize its effectiveness in scenarios such as high-speed data transmission and real-time signal processing.
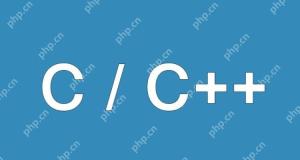
The delegate constructor in C is a function introduced by C 11, allowing one constructor to call another constructor of the same class. 1. It simplifies the writing of constructors and avoids code duplication. 2. This mechanism improves the clarity and maintainability of the code. 3. When using it, be careful to avoid loop calls, and the delegate call must be the first statement in the constructor body.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
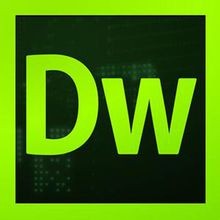
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
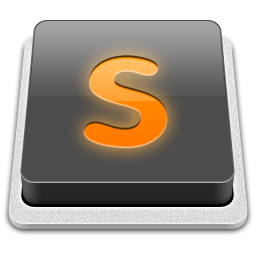
SublimeText3 Mac version
God-level code editing software (SublimeText3)
