Reading Numeric Data from a Text File in C
If you have a text file containing numeric data, you may want to read it and assign the numbers to variables in C for further processing. This can be achieved using C 's file handling capabilities.
To read numeric data from a text file, follow these steps:
-
Open the Text File:
ifstream myfile; myfile.open("data.txt");
-
Read the Data:
There are two main approaches to reading numeric data:-
Using Stream Extraction Operator (>>) Repeatedly:
float a; while (myfile >> a) { // Process the value of 'a' here... }
This method repeatedly reads numbers from the file until it reaches the end of file.
-
Reading Multiple Values Using Chained Extraction Operators:
float a, b, c, d, e, f; myfile >> a >> b >> c >> d >> e >> f;
This method reads multiple values in one go if you know the exact number of elements in the file.
-
-
Close the Text File:
myfile.close();
Handling Text Files with Spaces:
In the example text file mentioned in the problem, numbers are separated by spaces. To handle this, you can:
- Use the getline() function to read a line of text at a time and then extract the numbers using stringstream.
- Use a loop to iterate through the line and read the numbers using the stream extraction operator (>>).
Specific Value or Skipping Values:
If you need to read a specific value or skip values in the file, you can:
- For a Specific Value: Use a loop to read and discard values until you reach the desired location.
- For Skipping Values: Use a loop to skip a specified number of values before reading the desired data.
By following these steps, you can effectively read numeric data from a text file and assign it to variables in C .
The above is the detailed content of How to Read Numeric Data from a Text File in C ?. For more information, please follow other related articles on the PHP Chinese website!
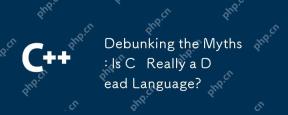
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
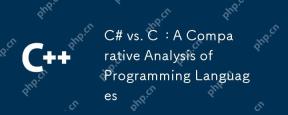
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
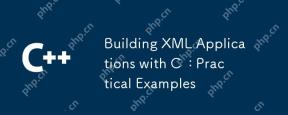
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
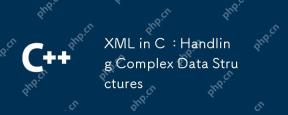
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
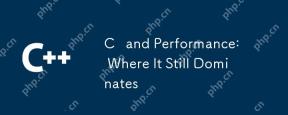
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
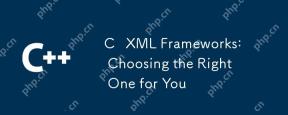
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
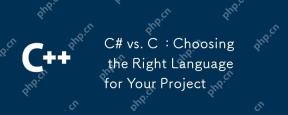
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
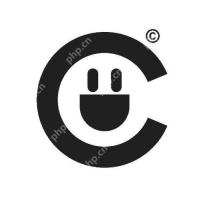
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
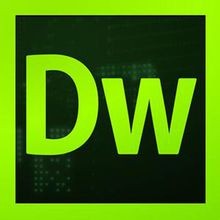
Dreamweaver CS6
Visual web development tools
