Comparing Version Strings in Java
Comparing version numbers is a common task in software development. However, it can be difficult to determine the correct ordering of versions that contain multiple components. Consider the following examples:
1.0 <p>A simple string comparison (e.g., compareTo()) is insufficient, as it doesn't account for the hierarchical nature of version numbers.</p><p><strong>A Comprehensive Solution</strong></p><p>To address this issue, we present a robust Java class that implements the Comparable interface to enable version number comparisons:</p><pre class="brush:php;toolbar:false">public class Version implements Comparable<version> { // ... (complete class definition) @Override public int compareTo(Version that) { // ... (implementation) } }</version>
The compareTo() method takes the following approach:
- Split both version strings into individual components using a dot (.) as the separator.
- Compare each corresponding component as an integer.
- If components are missing in one version, they are assumed to be zero.
- Return a negative, zero, or positive value based on the comparison results.
This method ensures that the version ordering follows the desired rules. For example, "1.0" is less than "1.1" because the major version number is lower.
Sample Usage
Version a = new Version("1.1"); Version b = new Version("1.1.1"); a.compareTo(b); // return -1 (a<b></b><p><strong>Customizable Behavior</strong></p><p>The matches() method in the constructor validates the format of the version string. This validation can be customized to meet the needs of specific scenarios.</p><p><strong>Note:</strong></p><p>It's important to be aware of potential pitfalls, such as the fact that "2.06" and "2.060" are considered different versions by this solution.</p>
The above is the detailed content of How Can You Effectively Compare Version Strings in Java?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
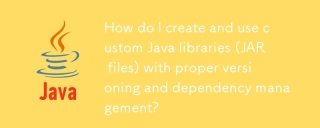
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
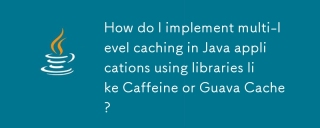
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
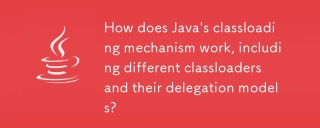
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
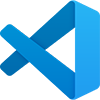
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
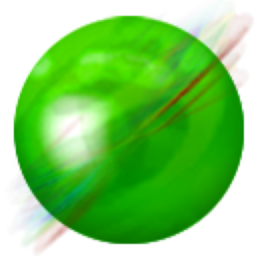
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
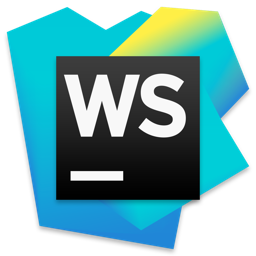
WebStorm Mac version
Useful JavaScript development tools