Performing Join Queries with Sequelize in Node.js
When working with Node.js and the Sequelize object-relational mapping (ORM), join queries can provide a powerful tool for efficiently retrieving related data from multiple tables. This article outlines how to execute join queries using Sequelize, addressing common scenarios.
Scenario: Retrieving Posts with Associated User Information
Let's consider two models, User and Post, representing users and their posts, respectively. To retrieve a list of posts along with their corresponding user information, you can use the findAll() method with the include option:
Posts.findAll({ include: [{ model: User, required: true }] }).then(posts => { // Access posts and associated user information... });
Setting required: true within the include option ensures that only posts with associated users are returned, effectively performing an inner join.
Scenario: Filtering Posts Based on User Attributes
To filter posts based on specific user attributes, such as birth year, you can use the where clause within the include option:
Posts.findAll({ include: [{ model: User, where: { year_birth: 1984 } }] }).then(posts => { // Access posts belonging to users born in 1984... });
Scenario: Left Outer Joins
If you wish to retrieve all posts, regardless of whether they have an associated user, you can use the required: false option within the include option, resulting in a left outer join:
Posts.findAll({ include: [{ model: User, required: false }] }).then(posts => { // Access all posts, including those without users... });
Complex Joins with Where Clauses
You can combine include and where clauses to perform more complex join operations. For instance, to retrieve posts with a specific name and belonging to a user born in the same year as the post, you can use the following query:
Posts.findAll({ where: { name: "Sunshine" }, include: [{ model: User, where: ["year_birth = post_year"] }] }).then(posts => { // Access posts with the name "Sunshine" and users with matching birth years... });
By following these guidelines, you can effectively perform join queries using Sequelize in Node.js to retrieve related data from multiple tables efficiently and elegantly.
The above is the detailed content of How to Perform Join Queries with Sequelize in Node.js?. For more information, please follow other related articles on the PHP Chinese website!

MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
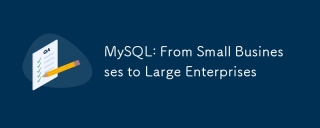
MySQL is suitable for small and large enterprises. 1) Small businesses can use MySQL for basic data management, such as storing customer information. 2) Large enterprises can use MySQL to process massive data and complex business logic to optimize query performance and transaction processing.
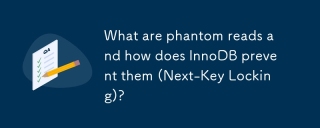
InnoDB effectively prevents phantom reading through Next-KeyLocking mechanism. 1) Next-KeyLocking combines row lock and gap lock to lock records and their gaps to prevent new records from being inserted. 2) In practical applications, by optimizing query and adjusting isolation levels, lock competition can be reduced and concurrency performance can be improved.
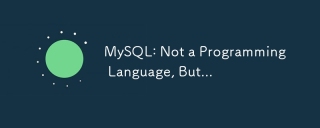
MySQL is not a programming language, but its query language SQL has the characteristics of a programming language: 1. SQL supports conditional judgment, loops and variable operations; 2. Through stored procedures, triggers and functions, users can perform complex logical operations in the database.
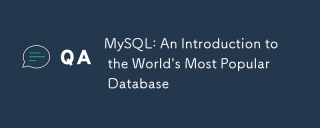
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
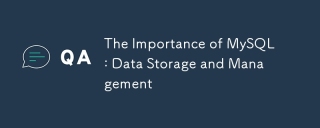
MySQL is an open source relational database management system suitable for data storage, management, query and security. 1. It supports a variety of operating systems and is widely used in Web applications and other fields. 2. Through the client-server architecture and different storage engines, MySQL processes data efficiently. 3. Basic usage includes creating databases and tables, inserting, querying and updating data. 4. Advanced usage involves complex queries and stored procedures. 5. Common errors can be debugged through the EXPLAIN statement. 6. Performance optimization includes the rational use of indexes and optimized query statements.
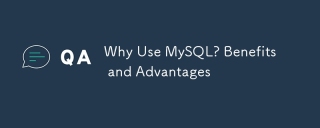
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
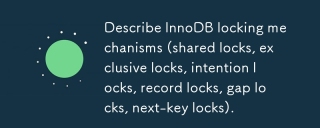
InnoDB's lock mechanisms include shared locks, exclusive locks, intention locks, record locks, gap locks and next key locks. 1. Shared lock allows transactions to read data without preventing other transactions from reading. 2. Exclusive lock prevents other transactions from reading and modifying data. 3. Intention lock optimizes lock efficiency. 4. Record lock lock index record. 5. Gap lock locks index recording gap. 6. The next key lock is a combination of record lock and gap lock to ensure data consistency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
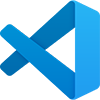
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
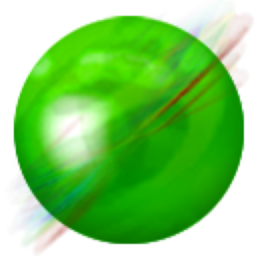
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
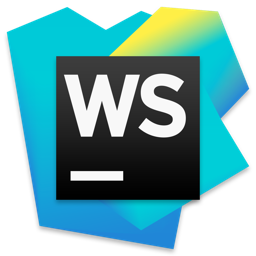
WebStorm Mac version
Useful JavaScript development tools