Read Numeric Data from a Text File in C
When working with numeric data stored in text files, it's crucial to know how to extract and assign the numbers to variables in your C program. This becomes especially important when dealing with formatted or unstructured data that may contain whitespace or multiple values in a single line.
Assigning First Number to Variable
Using ifstream to open the text file allows you to access the data stream and read the first number. However, to read subsequent numbers, you need a way to skip the white space that separates them.
Reading Multiple Numbers Using Loop
One approach to read multiple numbers is by utilizing loop statements. The following code demonstrates how to repeat the >> operator to read numbers from a text file until none remain:
#include <iostream> #include <fstream> using namespace std; int main() { std::fstream myfile("data.txt", std::ios_base::in); float a; while (myfile >> a) { printf("%f ", a); } getchar(); return 0; }</fstream></iostream>
Reading Multiple Numbers Using Chained >> Operator
If the number of elements in the file is known in advance, you can use the >> operator in sequence to read multiple numbers into separate variables:
#include <iostream> #include <fstream> using namespace std; int main() { std::fstream myfile("data.txt", std::ios_base::in); float a, b, c, d, e, f; myfile >> a >> b >> c >> d >> e >> f; printf("%f\t%f\t%f\t%f\t%f\t%f\n", a, b, c, d, e, f); getchar(); return 0; }</fstream></iostream>
Skipping Values in File
If you need to skip specific values in the file and read only a particular value, you can use a loop to advance the file position:
int skipped = 1233; for (int i = 0; i > tmp; } myfile >> value;
Alternative Approaches
Besides the aforementioned techniques, there is an array-based approach that can be used to read and store the numeric data. Additionally, you can find useful classes and solutions on platforms like GitHub to simplify this process further.
The above is the detailed content of How do I read numeric data from a text file in C ?. For more information, please follow other related articles on the PHP Chinese website!
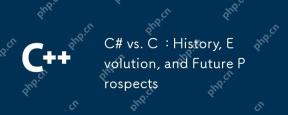
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
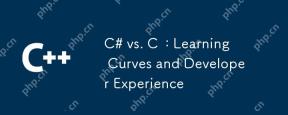
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
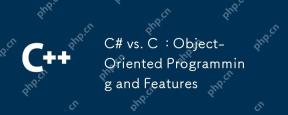
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
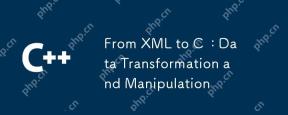
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
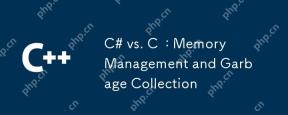
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
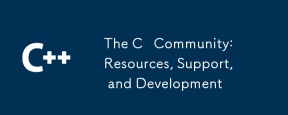
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
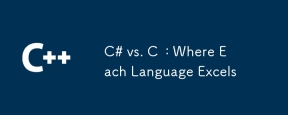
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
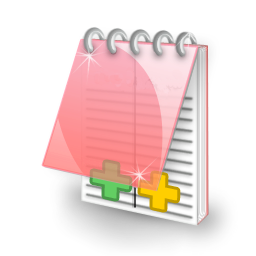
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool