Querying Network Connectivity with Python: How to Ping a Website or IP Address
In the world of networking, the ability to determine if a remote host is reachable is crucial. Python, a versatile programming language, provides a convenient means to perform ping operations, allowing you to verify network connectivity with ease.
Solution: Utilize Python's ICMP Socket Capabilities
To implement a ping utility in Python, you can leverage the ICMP (Internet Control Message Protocol) socket functionality provided by the operating system. As ICMP sockets require root privileges in Linux, it's important to note that your Python script may need to be run as root.
Sample Implementation
The following Python code snippet showcases how to ping a website or IP address using the ping module:
import ping try: ping.verbose_ping('www.google.com', count=3) delay = ping.Ping('www.wikipedia.org', timeout=2000).do() except socket.error as e: print("Ping Error:", e)
This code imports the ping module and attempts to ping a specified website or IP address. By default, it sends three ping requests, but you can customize this by adjusting the count parameter. Additionally, you can retrieve the delay (round-trip time) for a successful ping operation by calling Ping.do().
Diving into the Code
The ping module offers a comprehensive set of features. Exploring the source code of verbose_ping and Ping.do can provide valuable insights into implementing custom ping functionality.
By utilizing Python's powerful ICMP socket capabilities, you can effectively monitor network connectivity, troubleshoot connectivity issues, and gain valuable insights into your network infrastructure.
The above is the detailed content of How to Ping a Website or IP Address with Python?. For more information, please follow other related articles on the PHP Chinese website!
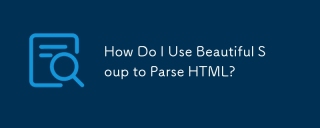
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
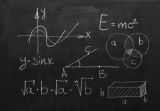
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
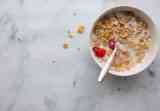
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
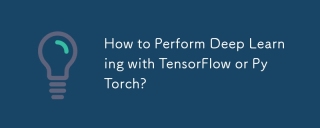
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
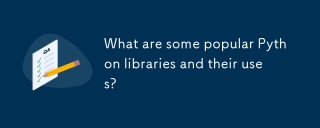
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
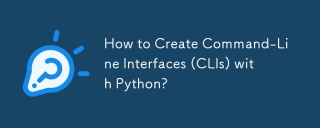
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
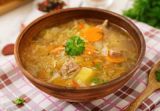
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
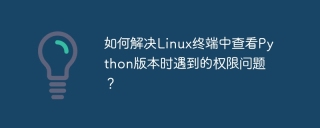
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
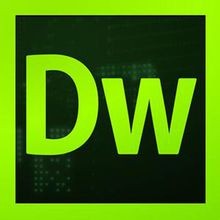
Dreamweaver CS6
Visual web development tools
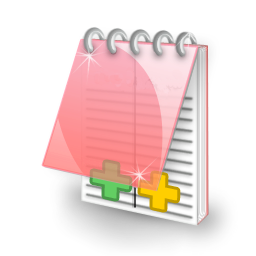
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
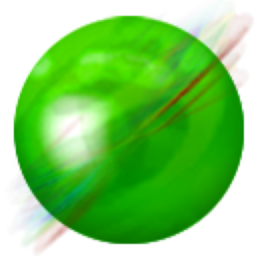
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
