Parsing JSON in Kotlin with Native Solutions
When processing deep JSON object strings, it's essential to efficiently parse and map them into custom classes. This guide discusses the native Kotlin approach to this task using the kotlinx.serialization library.
The kotlinx.serialization library is the recommended future approach for parsing in Kotlin. It provides a comprehensive solution for serializing and deserializing various types, including complex JSON objects.
Let's dive into a practical example:
import kotlinx.serialization.* import kotlinx.serialization.json.Json @Serializable data class MyModel(val a: Int, @Optional val b: String = "42") fun main(args: Array<string>) { // Serializing objects val jsonData = Json.encodeToString(MyModel.serializer(), MyModel(42)) println(jsonData) // { "a": 42, "b": "42" } // Serializing lists val jsonList = Json.encodeToString(MyModel.serializer().list, listOf(MyModel(42))) println(jsonList) // [{"a":42,"b":"42"}] // Parsing data back val obj = Json.decodeFromString(MyModel.serializer(), """ { "a": 42 }""") println(obj) // MyModel(a=42, b=null) }</string>
In this example, we:
- Define a Kotlin data class, MyModel, to represent the JSON structure.
- Use the Json.encodeToString method to serialize the object into a JSON string.
- Parse the JSON string back into a MyModel object using Json.decodeFromString.
- The @Serializable annotation indicates that the class can be serialized/deserialized.
- The @Optional annotation allows properties to have default values.
This approach provides a clean and effective way to parse JSON in Kotlin without external dependencies.
The above is the detailed content of How Can Kotlin\'s kotlinx.serialization Library Efficiently Parse JSON Data?. For more information, please follow other related articles on the PHP Chinese website!
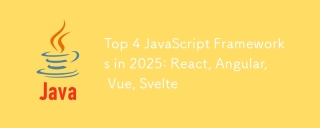
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
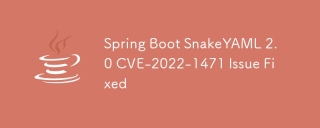
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
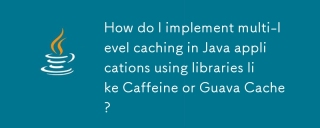
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
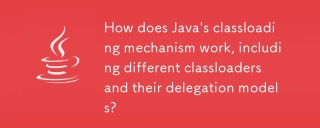
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
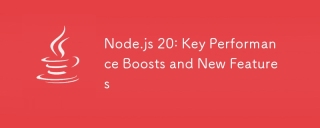
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
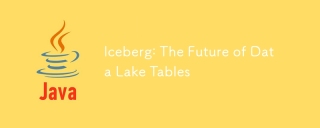
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
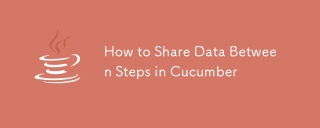
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
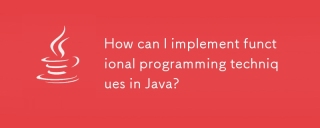
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
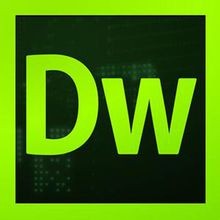
Dreamweaver CS6
Visual web development tools
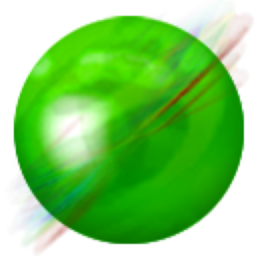
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
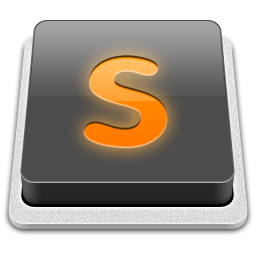
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
