Handling Passwords Securely in Source Code
In the context of accessing a RESTful API using basic authentication, storing the username and password in plaintext is a security risk. To enhance security, consider the following recommendations:
1. Convert Passwords to Character Arrays
Replace the plain text password with a character array. This prevents the use of String objects, which retain data after being set to null.
2. Encrypt Credentials and Decrypt Temporarily
Encrypt the credentials with an algorithm such as Triple Data Encryption Standard (3DES) before storing them. Decrypt them only during the authentication process.
3. Store Credentials Externally
Avoid hard-coding credentials. Instead, store them in a centralized location, such as a configuration file or database. Encrypt them before saving the file, and optionally apply another layer of encryption to the file itself.
4. Protect Transmission
Use Transport Layer Security (TLS) or Secure Sockets Layer (SSL) to secure the transmission process.
5. Obfuscate Code
Apply obfuscation techniques to your compiled code to conceal sensitive data.
Example of Password Encryption and Decryption:
The following code sample demonstrates the first and second steps outlined above:
import java.util.Arrays; import javax.crypto.Cipher; import javax.crypto.SecretKey; import javax.crypto.SecretKeyFactory; import javax.crypto.spec.PBEKeySpec; import javax.crypto.spec.PBEParameterSpec; public class PasswordEncryptionExample { private static final char[] PASSWORD = "Unauthorized_Personel_Is_Unauthorized".toCharArray(); private static final byte[] SALT = { (byte) 0xde, (byte) 0x33, (byte) 0x10, (byte) 0x12, (byte) 0xde, (byte) 0x33, (byte) 0x10, (byte) 0x12 }; public static void main(String[] args) throws Exception { String password = "LetMePass_Word"; char[] passwordArray = password.toCharArray(); SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("PBEWithMD5AndDES"); SecretKey key = keyFactory.generateSecret(new PBEKeySpec(PASSWORD)); Cipher pbeCipher = Cipher.getInstance("PBEWithMD5AndDES"); pbeCipher.init(Cipher.ENCRYPT_MODE, key, new PBEParameterSpec(SALT, 20)); byte[] encryptedPassword = pbeCipher.doFinal(passwordArray); // Cleanup password data sources Arrays.fill(passwordArray, (char) 0); Arrays.fill(encryptedPassword, (byte) 0); // Decrypt the encrypted password pbeCipher.init(Cipher.DECRYPT_MODE, key, new PBEParameterSpec(SALT, 20)); byte[] decryptedPassword = pbeCipher.doFinal(encryptedPassword); String decryptedPasswordString = new String(decryptedPassword); System.out.println("Decrypted password: " + decryptedPasswordString); } }
The above is the detailed content of How to Securely Handle Passwords in Source Code?. For more information, please follow other related articles on the PHP Chinese website!
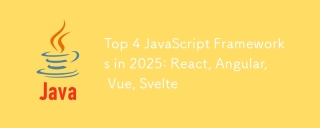
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
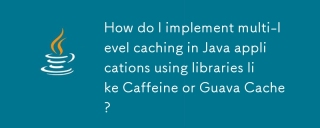
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
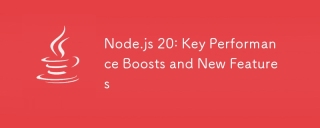
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
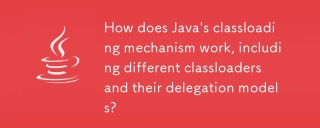
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
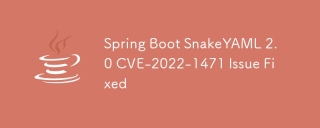
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
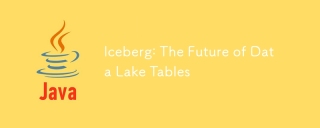
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
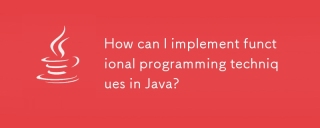
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
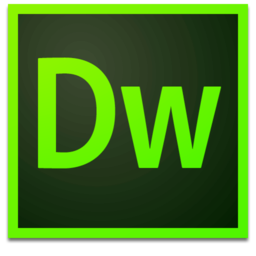
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
