Returns Undefined Dilemma: Unveiling the Mystery Behind Functions and Array Iterations
In the realm of programming, a common enigma arises when using functions and array iterations. While a return statement is often employed, the outcome may still be undefined. To uncover the reasons behind this perplexing issue, let's explore a specific case involving a function called getByKey that attempts to retrieve an object from an array based on a key.
The Enigma
The getByKey function, intended to search for an object in an array of objects, consistently returns undefined. Despite the presence of a return statement within its inner callback function, this enigmatic behavior has baffled developers.
Exploring the Resolution
Upon investigation, it becomes apparent that the return statement is executed within the callback function provided to the forEach method, which is not the same as returning from the getByKey function itself. To rectify this issue, we can either modify the code to utilize the map method instead of forEach, or we can opt for a traditional for loop, which offers more control over the iteration process.
Optimized Code with Map
Using the map method, we transform the array into a new array containing the desired results. For each element in the original array, we apply the callback function, which searches for the key and returns the matching object.
function getByKey(key) { return data.map(function (val) { if (val.Key === key) { return val; } }).filter(function (val) { return val !== undefined; })[0]; }
Simplified Solution with For Loop
For greater efficiency, we can employ a for loop to iterate over the array elements. This approach provides direct control over the loop and allows us to break out immediately when the matching object is found.
function getByKey(key) { for (var i = 0; i <p><strong>Understanding the Subtlety</strong></p><p>It's crucial to note that the callback function in forEach does not directly return from the containing function. The return statement within the callback affects the execution of the callback itself, but not the execution flow of the enclosing function. Therefore, it's essential to carefully consider the placement of return statements when working with array iteration functions.</p>
The above is the detailed content of Why Does My Function Return Undefined When Using Array Iterations?. For more information, please follow other related articles on the PHP Chinese website!
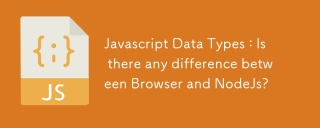
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
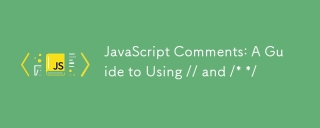
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
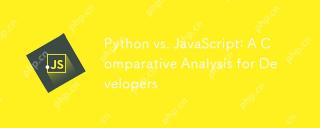
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
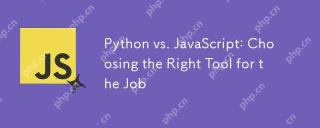
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
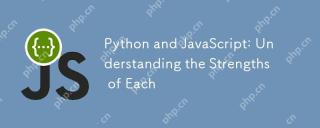
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
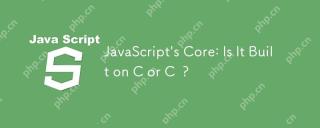
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
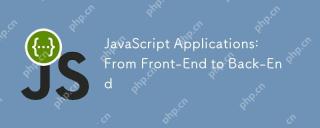
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
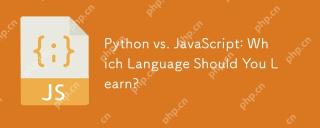
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use
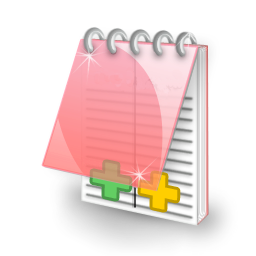
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor
